Running Parameterized FCNNs#
Now that we have taken time to explore and understand FCNNs, we will now run a parameterization of our own.
# Import required modules
import numpy as np
import xarray as xr
import matplotlib.pyplot as plt
import pyqg
import json
import fsspec
from pyqg_parameterization_benchmarks.neural_networks import FCNNParameterization, FullyCNN
%matplotlib inline
Below we are loading a sample dataset, as training data for our parameterization, of a high-resolution simulation in the eddy configuration started from random noise and run over a span of 10 simulation years that has been coarsened using Operator 2 (a spectral truncation + softer Gaussian filter).
training_ds = xr.open_zarr('/home/jovyan/datasets/eddy/op2/ds1').load()
training_ds
<xarray.Dataset> Dimensions: (time: 87, lev: 2, y: 64, x: 64, l: 64, k: 33, lev_mid: 1) Coordinates: * k (k) float64 0.0 6.283e-06 ... 0.0001948 0.0002011 * l (l) float64 0.0 6.283e-06 ... -1.257e-05 -6.283e-06 * lev (lev) int64 1 2 * lev_mid (lev_mid) float64 1.5 * time (time) float64 0.0 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0 * x (x) float64 7.812e+03 2.344e+04 ... 9.766e+05 9.922e+05 * y (y) float64 7.812e+03 2.344e+04 ... 9.766e+05 9.922e+05 Data variables: (12/23) Qy (time, lev) float64 1.039e-10 -7.222e-12 ... -7.222e-12 Ubg (time, lev) float64 0.025 0.0 0.025 0.0 ... 0.0 0.025 0.0 dqdt (time, lev, y, x) float64 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 dqhdt (time, lev, l, k) complex128 0j 0j 0j 0j ... 0j 0j 0j 0j p (time, lev, y, x) float64 -39.07 -62.7 ... -77.82 33.89 ph (time, lev, l, k) complex128 0j ... (11.85916120645425... ... ... v (time, lev, y, x) float64 -0.001415 ... 0.006776 v_subgrid_forcing (time, lev, y, x) float64 2.498e-13 ... 8.655e-11 vfull (time, lev, y, x) float64 -0.001415 ... 0.006776 vh (time, lev, l, k) complex128 0j ... 0.007440851489267999j vq_subgrid_flux (time, lev, y, x) float64 -2.542e-12 ... 1.934e-10 vv_subgrid_flux (time, lev, y, x) float64 3.388e-07 ... 1.838e-06 Attributes: (12/23) pyqg:L: 1000000.0 pyqg:M: 4096 pyqg:W: 1000000.0 pyqg:beta: 1.5e-11 pyqg:del2: 0.8 pyqg:delta: 0.25 ... ... pyqg:tavestart: 315360000.0 pyqg:tc: 0 pyqg:tmax: 1576800000.0 pyqg:twrite: 1000.0 reference: https://pyqg.readthedocs.io/en/latest/index.html title: pyqg: Python Quasigeostrophic Model
- time: 87
- lev: 2
- y: 64
- x: 64
- l: 64
- k: 33
- lev_mid: 1
- k(k)float640.0 6.283e-06 ... 0.0002011
- long_name :
- spectal space grid points in the k direction
- units :
- zonal wavenumber
array([0.000000e+00, 6.283185e-06, 1.256637e-05, 1.884956e-05, 2.513274e-05, 3.141593e-05, 3.769911e-05, 4.398230e-05, 5.026548e-05, 5.654867e-05, 6.283185e-05, 6.911504e-05, 7.539822e-05, 8.168141e-05, 8.796459e-05, 9.424778e-05, 1.005310e-04, 1.068142e-04, 1.130973e-04, 1.193805e-04, 1.256637e-04, 1.319469e-04, 1.382301e-04, 1.445133e-04, 1.507964e-04, 1.570796e-04, 1.633628e-04, 1.696460e-04, 1.759292e-04, 1.822124e-04, 1.884956e-04, 1.947787e-04, 2.010619e-04])
- l(l)float640.0 6.283e-06 ... -6.283e-06
- long_name :
- spectal space grid points in the l direction
- units :
- meridional wavenumber
array([ 0.000000e+00, 6.283185e-06, 1.256637e-05, 1.884956e-05, 2.513274e-05, 3.141593e-05, 3.769911e-05, 4.398230e-05, 5.026548e-05, 5.654867e-05, 6.283185e-05, 6.911504e-05, 7.539822e-05, 8.168141e-05, 8.796459e-05, 9.424778e-05, 1.005310e-04, 1.068142e-04, 1.130973e-04, 1.193805e-04, 1.256637e-04, 1.319469e-04, 1.382301e-04, 1.445133e-04, 1.507964e-04, 1.570796e-04, 1.633628e-04, 1.696460e-04, 1.759292e-04, 1.822124e-04, 1.884956e-04, 1.947787e-04, -2.010619e-04, -1.947787e-04, -1.884956e-04, -1.822124e-04, -1.759292e-04, -1.696460e-04, -1.633628e-04, -1.570796e-04, -1.507964e-04, -1.445133e-04, -1.382301e-04, -1.319469e-04, -1.256637e-04, -1.193805e-04, -1.130973e-04, -1.068142e-04, -1.005310e-04, -9.424778e-05, -8.796459e-05, -8.168141e-05, -7.539822e-05, -6.911504e-05, -6.283185e-05, -5.654867e-05, -5.026548e-05, -4.398230e-05, -3.769911e-05, -3.141593e-05, -2.513274e-05, -1.884956e-05, -1.256637e-05, -6.283185e-06])
- lev(lev)int641 2
- long_name :
- vertical levels
array([1, 2])
- lev_mid(lev_mid)float641.5
- long_name :
- vertical level interface
array([1.5])
- time(time)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- long_name :
- model time
- units :
- s
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
- x(x)float647.812e+03 2.344e+04 ... 9.922e+05
- long_name :
- real space grid points in the x direction
- units :
- grid point
array([ 7812.5, 23437.5, 39062.5, 54687.5, 70312.5, 85937.5, 101562.5, 117187.5, 132812.5, 148437.5, 164062.5, 179687.5, 195312.5, 210937.5, 226562.5, 242187.5, 257812.5, 273437.5, 289062.5, 304687.5, 320312.5, 335937.5, 351562.5, 367187.5, 382812.5, 398437.5, 414062.5, 429687.5, 445312.5, 460937.5, 476562.5, 492187.5, 507812.5, 523437.5, 539062.5, 554687.5, 570312.5, 585937.5, 601562.5, 617187.5, 632812.5, 648437.5, 664062.5, 679687.5, 695312.5, 710937.5, 726562.5, 742187.5, 757812.5, 773437.5, 789062.5, 804687.5, 820312.5, 835937.5, 851562.5, 867187.5, 882812.5, 898437.5, 914062.5, 929687.5, 945312.5, 960937.5, 976562.5, 992187.5])
- y(y)float647.812e+03 2.344e+04 ... 9.922e+05
- long_name :
- real space grid points in the y direction
- units :
- grid point
array([ 7812.5, 23437.5, 39062.5, 54687.5, 70312.5, 85937.5, 101562.5, 117187.5, 132812.5, 148437.5, 164062.5, 179687.5, 195312.5, 210937.5, 226562.5, 242187.5, 257812.5, 273437.5, 289062.5, 304687.5, 320312.5, 335937.5, 351562.5, 367187.5, 382812.5, 398437.5, 414062.5, 429687.5, 445312.5, 460937.5, 476562.5, 492187.5, 507812.5, 523437.5, 539062.5, 554687.5, 570312.5, 585937.5, 601562.5, 617187.5, 632812.5, 648437.5, 664062.5, 679687.5, 695312.5, 710937.5, 726562.5, 742187.5, 757812.5, 773437.5, 789062.5, 804687.5, 820312.5, 835937.5, 851562.5, 867187.5, 882812.5, 898437.5, 914062.5, 929687.5, 945312.5, 960937.5, 976562.5, 992187.5])
- Qy(time, lev)float641.039e-10 -7.222e-12 ... -7.222e-12
- long_name :
- background potential vorticity gradient
- units :
- m^-1 s^-1
array([[ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], ... [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12], [ 1.03888889e-10, -7.22222222e-12]])
- Ubg(time, lev)float640.025 0.0 0.025 ... 0.0 0.025 0.0
- long_name :
- background zonal velocity
- units :
- m s^-1
array([[0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], ... [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ], [0.025, 0. ]])
- dqdt(time, lev, y, x)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- long_name :
- previous partial derivative of potential vorticity wrt. time in real space
- units :
- s^-2
array([[[[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]]], [[[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ... [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]]], [[[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]]]])
- dqhdt(time, lev, l, k)complex1280j 0j 0j 0j 0j ... 0j 0j 0j 0j 0j
- long_name :
- previous partial derivative of potential vorticity wrt. time in spectral space
- units :
- s^-2
array([[[[0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], ..., [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j]], [[0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], ..., [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j]]], [[[0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], ... [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j]]], [[[0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], ..., [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j]], [[0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], ..., [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j], [0.+0.j, 0.+0.j, 0.+0.j, ..., 0.+0.j, 0.+0.j, 0.+0.j]]]])
- p(time, lev, y, x)float64-39.07 -62.7 ... -77.82 33.89
- long_name :
- streamfunction in real space
- units :
- m^2 s^-1
array([[[[-3.90744176e+01, -6.27024853e+01, -9.00949080e+01, ..., 2.52882694e+01, 1.29117233e+00, -1.76390254e+01], [-3.85156603e+01, -6.21161562e+01, -8.94866933e+01, ..., 2.52572007e+01, 1.61500254e+00, -1.71440203e+01], [-3.81484423e+01, -6.21442310e+01, -8.95385318e+01, ..., 2.53293267e+01, 2.04528352e+00, -1.65730736e+01], ..., [-3.90295458e+01, -6.27486814e+01, -9.00719932e+01, ..., 2.55282350e+01, 1.10107929e+00, -1.76704415e+01], [-3.93522830e+01, -6.30348355e+01, -9.03435919e+01, ..., 2.55458504e+01, 1.01324149e+00, -1.79087409e+01], [-3.93739107e+01, -6.31023985e+01, -9.03988204e+01, ..., 2.54454393e+01, 1.09414228e+00, -1.78800100e+01]], [[-4.35464961e+01, -6.78399888e+01, -9.32551198e+01, ..., 2.51513778e+01, 2.39539493e+00, -2.02783853e+01], [-4.33542944e+01, -6.76514746e+01, -9.30770356e+01, ..., 2.52252454e+01, 2.53273909e+00, -2.00992868e+01], [-4.31505967e+01, -6.74743180e+01, -9.29191251e+01, ..., 2.53502705e+01, 2.71177902e+00, -1.98891307e+01], ... [ 8.46562974e+02, 1.41762563e+03, 1.68828477e+03, ..., -4.36238486e+02, -3.04609918e+02, 1.41707966e+02], [ 1.13175818e+03, 1.42140294e+03, 1.36657315e+03, ..., -2.56747339e+02, 1.23397676e+02, 6.00647396e+02], [ 1.10944082e+03, 8.60367040e+02, 3.56030688e+02, ..., -1.57768202e+02, 5.48675345e+02, 9.94105875e+02]], [[ 1.82208324e+02, 1.51755466e+02, 6.77539493e+01, ..., -1.03289012e+02, 3.19351415e+01, 1.38946785e+02], [ 1.91495143e+02, 7.44986527e+01, -7.96508070e+01, ..., 2.74527688e+01, 1.60892286e+02, 2.24547364e+02], [ 1.46211400e+02, -2.52635028e+01, -2.04663034e+02, ..., 1.78888870e+02, 2.64624049e+02, 2.54487801e+02], ..., [ 1.11286953e+01, 1.18801994e+02, 2.00058561e+02, ..., -2.53751828e+02, -2.11095393e+02, -1.11127369e+02], [ 6.48618912e+01, 1.61769275e+02, 2.21488429e+02, ..., -2.25548253e+02, -1.60703167e+02, -5.39800253e+01], [ 1.24219323e+02, 1.70239073e+02, 1.68823669e+02, ..., -1.80501347e+02, -7.78188481e+01, 3.38855203e+01]]]])
- ph(time, lev, l, k)complex1280j ... (11.859161206454257+8.324...
- long_name :
- streamfunction in spectral space
- units :
- m^2 s^-1
array([[[[ 0.00000000e+00+0.00000000e+00j, -7.76933919e+04+3.79130465e+05j, -3.05610213e+04+2.96934595e+04j, ..., -1.03563182e+02-2.81097683e+02j, -1.03040988e+02-3.67318603e+02j, 4.57326806e+02+0.00000000e+00j], [-7.88568979e+02-1.16619076e+03j, -1.60391606e+02+5.03702711e+01j, -9.19441947e+01-2.90505614e+02j, ..., 7.06053958e-01+1.26330043e+00j, 2.69277193e+00-1.39867962e+00j, -1.11044597e+00-7.55703433e-01j], [ 5.55847381e+02-2.74180642e+02j, -4.30145609e+02+2.88173006e+02j, -1.30088874e+02+4.31714697e+01j, ..., -1.54334422e-01+1.30817730e+00j, 1.26591859e+00-1.15362174e+00j, 1.45376489e+00+3.00232697e-01j], ..., [ 1.47838418e+01+1.43290955e+01j, ... -6.97250580e+00+2.30848387e+01j], ..., [-6.29261260e+04-6.89159834e+04j, -4.76643724e+04-8.42219190e+03j, -3.71361588e+04-6.19506625e+04j, ..., 2.12852246e+01+5.69942638e+01j, -3.40940187e+01+5.73216676e+01j, -9.27974254e+00-2.88910591e+00j], [ 1.42741196e+05+6.51045879e+04j, -9.95800283e+04-4.45710459e+04j, -1.51727739e+04+2.58436915e+04j, ..., 2.97599159e+00-1.57740551e+01j, 4.28016735e+01-4.42485939e+01j, -6.97250580e+00-2.30848387e+01j], [-3.61697673e+05-2.30222379e+05j, -4.34722081e+04+2.57141593e+04j, 1.05292759e+05+2.83448289e+04j, ..., 2.53452960e+01-2.28966294e+01j, -6.40000612e+01+1.86472482e+01j, 1.18591612e+01+8.32476912e+00j]]]])
- q(time, lev, y, x)float645.345e-07 5.202e-07 ... 8.391e-07
- long_name :
- potential vorticity in real space
- units :
- s^-1
array([[[[ 5.34469755e-07, 5.20241925e-07, 5.27929446e-07, ..., 5.75679661e-07, 5.84055283e-07, 5.31119355e-07], [ 5.30827986e-07, 5.14545980e-07, 5.19037063e-07, ..., 5.77156443e-07, 5.82651152e-07, 5.29255736e-07], [ 5.28650473e-07, 5.22002855e-07, 5.24450952e-07, ..., 5.78620086e-07, 5.78298757e-07, 5.24305611e-07], ..., [ 5.35570625e-07, 5.23105227e-07, 5.25177791e-07, ..., 5.74086187e-07, 5.88362881e-07, 5.32950465e-07], [ 5.34080271e-07, 5.20854420e-07, 5.25581625e-07, ..., 5.74568037e-07, 5.87434058e-07, 5.30823813e-07], [ 5.35096862e-07, 5.23572328e-07, 5.27279593e-07, ..., 5.74047866e-07, 5.86231705e-07, 5.31326609e-07]], [[ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], ... [-3.71157006e-06, -7.39131313e-06, -9.36708707e-06, ..., 1.88592615e-06, 2.87698618e-06, 1.23993870e-06], [-5.53062962e-06, -7.79550374e-06, -7.88717518e-06, ..., 6.11646181e-07, 8.05275813e-08, -1.41639166e-06], [-6.80822172e-06, -4.21373219e-06, -6.17164494e-07, ..., 2.66558469e-07, -4.56054109e-06, -6.64128078e-06]], [[-1.28890644e-07, -6.27935902e-07, -1.02881977e-06, ..., 3.23030291e-07, 5.22186208e-07, 3.24050658e-07], [-6.44588560e-07, -1.02626266e-06, -1.35916353e-06, ..., 1.68397278e-07, 3.80673477e-08, -3.02722586e-07], [-4.83605082e-07, -1.06873218e-06, -1.51858422e-06, ..., -1.62658547e-07, -1.86683811e-07, -2.09784644e-07], ..., [ 5.53551010e-07, 7.73233363e-07, 7.39899666e-07, ..., 7.97735452e-08, 2.19056995e-07, 3.17040824e-07], [ 8.89678105e-07, 8.31479207e-07, 5.46062958e-07, ..., 2.34721981e-07, 5.82553452e-07, 7.80516802e-07], [ 6.99250055e-07, 3.26306054e-07, -1.42610014e-07, ..., 3.11917621e-07, 6.96027278e-07, 8.39110908e-07]]]])
- q_forcing_total(time, lev, y, x)float646.654e-15 -6.717e-15 ... -2.717e-14
array([[[[ 6.65374236e-15, -6.71686306e-15, 6.81473034e-15, ..., -6.85414306e-15, 6.82208578e-15, -6.74760033e-15], [ 6.56288986e-15, -6.41203869e-15, 6.52544690e-15, ..., -6.92806855e-15, 6.71070030e-15, -6.59426876e-15], [ 7.51457268e-15, -7.48960868e-15, 7.35350740e-15, ..., -7.77556286e-15, 7.39097407e-15, -7.55323391e-15], ..., [ 6.80765282e-15, -6.94205855e-15, 6.77742386e-15, ..., -6.74205515e-15, 7.02603812e-15, -6.85950015e-15], [ 6.70350622e-15, -6.81771133e-15, 6.77368133e-15, ..., -7.01947403e-15, 6.65193000e-15, -6.64845543e-15], [ 6.77092240e-15, -6.77383052e-15, 6.93897269e-15, ..., -6.89961004e-15, 6.65046738e-15, -6.76066709e-15]], [[ 1.06197448e-18, -1.06197448e-18, 1.06197448e-18, ..., -1.06197448e-18, 1.06197448e-18, -1.06197448e-18], [ 1.06563757e-18, -1.06563757e-18, 1.06563757e-18, ..., -1.06563757e-18, 1.06563757e-18, -1.06563757e-18], [ 1.14739540e-18, -1.14739540e-18, 1.14739540e-18, ..., -1.14739540e-18, 1.14739540e-18, -1.14739540e-18], ... [-8.39116741e-13, -1.21297362e-12, 4.53914085e-12, ..., 1.79664261e-12, 4.91463278e-13, -2.18637520e-12], [ 4.85376773e-13, -1.36713797e-12, -1.85083061e-12, ..., -2.99839430e-12, 3.86963339e-12, -1.35145179e-12], [ 2.65992110e-12, 2.57396140e-12, 2.95532096e-13, ..., 3.21235769e-13, 9.74969762e-13, 3.95551533e-14]], [[ 5.95180074e-14, 6.43742619e-14, 4.74276292e-14, ..., -1.89956366e-14, -5.83464164e-14, 1.78033935e-16], [ 8.68602260e-14, 7.51438268e-14, -7.37129706e-15, ..., 6.26744366e-15, 4.84696060e-14, 1.09378079e-13], [-2.89995700e-14, -6.36563671e-14, 2.14767026e-14, ..., 3.57854004e-14, 6.96314073e-14, 7.87368004e-14], ..., [-6.17892457e-15, 1.48340887e-14, 2.93157660e-14, ..., -2.93820714e-14, -1.12462976e-14, -7.70683476e-15], [-2.82788697e-14, -2.56383278e-14, -3.40548476e-14, ..., 1.78586425e-14, -1.95306124e-14, 2.65787942e-15], [-8.53703815e-14, -4.33565915e-14, -3.43972259e-14, ..., -1.26872922e-14, 6.55230743e-15, -2.71725045e-14]]]])
- q_subgrid_forcing(time, lev, y, x)float641.738e-16 -1.107e-16 ... 2.716e-14
array([[[[ 1.73814937e-16, -1.10694236e-16, 1.28269646e-17, ..., 2.65857600e-17, 5.47152012e-18, -7.99569694e-17], [ 8.58101284e-17, -2.36661305e-16, 1.23253091e-16, ..., 2.79368555e-16, -6.20003102e-17, -5.44312294e-17], [-1.15939798e-16, 9.09758016e-17, 4.51254762e-17, ..., 3.76929979e-16, 7.65880990e-18, 1.54601036e-16], ..., [ 1.45310675e-16, -1.09049515e-17, 1.75539634e-16, ..., -2.10908346e-16, -7.30746234e-17, -9.34633447e-17], [ 3.09620192e-17, 8.32430937e-17, -3.92130941e-17, ..., 2.85005798e-16, 8.25382336e-17, -8.60128053e-17], [ 1.00841526e-17, -7.17603000e-18, -1.57966136e-16, ..., 1.18603486e-16, 1.30539178e-16, -2.03394655e-17]], [[ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], ... [ 6.93294028e-13, 1.35879633e-12, -4.68496357e-12, ..., -1.65081989e-12, -6.37285991e-13, 2.33219792e-12], [-5.57045198e-13, 1.43880640e-12, 1.77916219e-12, ..., 3.07006273e-12, -3.94130181e-12, 1.42312021e-12], [-2.71610391e-12, -2.51777858e-12, -3.51714914e-13, ..., -2.65052951e-13, -1.03115258e-12, 1.66276647e-14]], [[-5.94721728e-14, -6.44200965e-14, -4.73817946e-14, ..., 1.89498020e-14, 5.83922510e-14, -2.23868550e-16], [-8.67840202e-14, -7.52200326e-14, 7.44750285e-15, ..., -6.34364946e-15, -4.83934002e-14, -1.09454284e-13], [ 2.90976691e-14, 6.35582681e-14, -2.13786035e-14, ..., -3.58834995e-14, -6.95333083e-14, -7.88348994e-14], ..., [ 6.17008940e-15, -1.48252536e-14, -2.93246011e-14, ..., 2.93909066e-14, 1.12374625e-14, 7.71566993e-15], [ 2.82917427e-14, 2.56254549e-14, 3.40677205e-14, ..., -1.78715155e-14, 1.95434854e-14, -2.67075239e-15], [ 8.53862012e-14, 4.33407718e-14, 3.44130456e-14, ..., 1.26714725e-14, -6.53648773e-15, 2.71566848e-14]]]])
- qh(time, lev, l, k)complex128(0.002273901909224313+0j) ... (-...
- long_name :
- potential vorticity in spectral space
- units :
- s^-1
array([[[[ 2.27390191e-03+0.00000000e+00j, 1.48143334e-05-7.22914132e-05j, 2.12179403e-05-2.06156086e-05j, ..., 4.03889690e-06+1.09626272e-05j, 4.26722787e-06+1.52117348e-05j, -2.00789139e-05+0.00000000e+00j], [ 1.50361871e-07+2.22365613e-07j, 5.91875482e-08-1.85875864e-08j, 7.75535572e-08+2.45037153e-07j, ..., -2.75635864e-08-4.93178887e-08j, -1.11622069e-07+5.79787361e-08j, 4.87980026e-08+3.32090161e-08j], [-3.85914347e-07+1.90358445e-07j, 3.62821407e-07-2.43069633e-07j, 1.62344233e-07-5.38757768e-08j, ..., 6.04337164e-09-5.12251349e-08j, -5.26257046e-08+4.79573943e-08j, -6.40574783e-08-1.32292020e-08j], ..., [-2.02637520e-08-1.96404453e-08j, ... -4.85011888e-08-8.27544402e-07j], ..., [-1.28548503e-05-7.95871272e-05j, 1.20450190e-04-2.91633371e-04j, 2.75999860e-05-1.01481958e-04j, ..., -1.07762636e-06-1.21812955e-06j, 9.80093216e-07-1.84318686e-06j, 2.36931810e-07-3.90757884e-07j], [-3.63153140e-05+2.10262291e-04j, -1.01398654e-05-1.85242258e-04j, 2.16414291e-05+1.01006554e-04j, ..., -3.90634868e-07-3.18197806e-07j, -1.16225570e-06+1.88693689e-06j, -4.85011888e-08+8.27544402e-07j], [ 5.22662055e-05+5.59494992e-06j, 3.78815445e-05+9.05679058e-06j, 6.79350458e-05-9.69258840e-05j, ..., -5.74913083e-07+1.01308617e-06j, 2.35601699e-06-1.42181063e-06j, -2.15506361e-07-1.50214243e-07j]]]])
- u(time, lev, y, x)float64-3.197e-05 -4.684e-05 ... -0.006472
- long_name :
- zonal velocity anomaly
- units :
- m s^-1
array([[[[-3.19729253e-05, -4.68408357e-05, -4.31546865e-05, ..., 8.76839596e-06, -1.38703431e-05, -2.35121678e-05], [-3.41140132e-05, -1.56391398e-05, -1.77418332e-05, ..., -4.07581784e-06, -2.91243288e-05, -3.89325632e-05], [-1.16724934e-05, 6.67372962e-06, 9.85891083e-06, ..., -4.97932966e-06, -1.91656499e-05, -2.76029910e-05], ..., [ 4.55764669e-05, 4.25917396e-05, 3.36541965e-05, ..., -6.87900971e-06, 1.37794692e-05, 3.78278155e-05], [ 5.04171353e-06, 3.09379250e-06, 3.18541154e-06, ..., 4.12373241e-06, 2.65761279e-08, 1.91418052e-06], [-5.23994400e-06, -1.01006781e-06, 1.97875706e-06, ..., 8.59344609e-06, -1.06620761e-05, -7.15823464e-06]], [[-1.07791525e-05, -1.12762938e-05, -1.09048891e-05, ..., -3.03103984e-06, -6.97900310e-06, -9.55405245e-06], [-1.31395421e-05, -1.19425737e-05, -1.09475818e-05, ..., -6.44635932e-06, -1.04541963e-05, -1.29534313e-05], [-1.27915349e-05, -1.11171401e-05, -9.76481528e-06, ..., -9.51691022e-06, -1.21331660e-05, -1.35229631e-05], ... [-2.23975117e-02, -1.73844151e-02, -8.40325983e-03, ..., -1.09173911e-02, -2.21259147e-02, -2.54291988e-02], [-1.27167741e-02, 1.79871125e-02, 4.87550382e-02, ..., -1.03071771e-02, -3.15528991e-02, -3.24883450e-02], [ 1.88557079e-02, 5.07073251e-02, 7.29083413e-02, ..., -2.08458583e-03, -1.78244871e-02, -1.10257278e-02]], [[-2.69217144e-03, 2.86237508e-03, 8.24855009e-03, ..., -6.52199637e-03, -7.81110359e-03, -6.59392472e-03], [ 1.64599549e-03, 6.61193633e-03, 9.76250040e-03, ..., -9.89275327e-03, -8.16499031e-03, -3.86577442e-03], [ 3.17502149e-03, 4.99373977e-03, 5.28557787e-03, ..., -8.36652542e-03, -4.57271920e-03, -2.80895870e-04], ..., [-3.89758561e-03, -4.63884114e-03, -4.63652124e-03, ..., -1.48931238e-03, -2.48091221e-03, -3.13928397e-03], [-3.40594151e-03, -1.25105065e-03, 1.54065687e-03, ..., -2.23361267e-03, -4.23543444e-03, -4.57318246e-03], [-4.12094602e-03, 3.14988155e-05, 4.85938486e-03, ..., -3.67632372e-03, -6.24166405e-03, -6.47178273e-03]]]])
- u_subgrid_forcing(time, lev, y, x)float64-1.158e-14 1.335e-13 ... 1.938e-10
array([[[[-1.15784057e-14, 1.33472894e-13, -3.83026104e-13, ..., 2.65187145e-13, -1.40905680e-13, -2.87763798e-13], [-3.54642897e-14, 3.30561806e-13, -1.98851868e-13, ..., 3.82866240e-13, 1.54503273e-13, -5.86425950e-15], [-2.50606672e-14, -4.60461852e-13, 1.04289406e-13, ..., 6.79057584e-13, 3.82016914e-15, -4.32553711e-14], ..., [-5.16349635e-14, -3.17744006e-13, -1.78991583e-13, ..., 1.15387602e-12, 5.44229674e-13, -1.99630655e-13], [-1.25469998e-13, -1.27955263e-13, -3.63304037e-13, ..., -8.89694978e-13, -4.26512647e-13, -2.53900205e-14], [ 2.14996132e-13, 2.36533992e-13, 5.76893913e-13, ..., -6.63521880e-13, 3.22014055e-14, 1.68705141e-13]], [[-2.11333510e-15, -1.25347480e-15, -2.01789041e-15, ..., -8.31752635e-16, -1.67471146e-15, -4.68720623e-16], [-2.56788490e-15, -7.55753489e-16, -5.15914009e-16, ..., 1.88110201e-15, 7.46213673e-16, 4.47594149e-16], [ 1.20698658e-15, -6.07357331e-16, 1.25238744e-16, ..., -1.09502041e-15, -1.15284648e-16, -2.20684644e-15], ... [ 3.12702691e-09, -2.65319151e-09, 2.37835670e-08, ..., -1.05045163e-08, -4.78436762e-09, 1.62768073e-08], [-6.84969960e-09, 8.03317652e-09, -2.08253401e-08, ..., 1.03682370e-08, 1.05851124e-08, -2.10181226e-08], [-5.72654263e-09, 8.01902727e-09, 4.46308265e-08, ..., -1.43702303e-08, 1.76638578e-08, 1.44538543e-08]], [[-2.66067270e-10, -1.26819930e-10, -1.12966976e-10, ..., 3.96591471e-11, -1.66628484e-10, -5.10335403e-10], [ 3.23736239e-10, 2.57064402e-10, 2.89838427e-10, ..., -5.98393159e-10, -4.16067043e-10, 1.29324992e-10], [ 7.67291538e-10, 5.53339773e-10, -7.98493677e-12, ..., 2.19097568e-10, 9.34078594e-11, 5.45946698e-10], ..., [ 1.13835832e-10, -5.69792694e-11, 9.60986533e-11, ..., -3.03869513e-10, -1.36690338e-10, 2.62327566e-10], [-1.18505451e-10, 3.72686696e-10, 4.56342566e-10, ..., 1.23999209e-10, 7.24612727e-11, -2.69663448e-10], [-9.12817214e-11, -4.15721288e-10, -2.70819394e-10, ..., -4.47965355e-10, -1.82078435e-10, 1.93840735e-10]]]])
- ufull(time, lev, y, x)float640.02497 0.02495 ... -0.006472
- long_name :
- zonal full velocities in real space
- units :
- m s^-1
array([[[[ 2.49680271e-02, 2.49531592e-02, 2.49568453e-02, ..., 2.50087684e-02, 2.49861297e-02, 2.49764878e-02], [ 2.49658860e-02, 2.49843609e-02, 2.49822582e-02, ..., 2.49959242e-02, 2.49708757e-02, 2.49610674e-02], [ 2.49883275e-02, 2.50066737e-02, 2.50098589e-02, ..., 2.49950207e-02, 2.49808344e-02, 2.49723970e-02], ..., [ 2.50455765e-02, 2.50425917e-02, 2.50336542e-02, ..., 2.49931210e-02, 2.50137795e-02, 2.50378278e-02], [ 2.50050417e-02, 2.50030938e-02, 2.50031854e-02, ..., 2.50041237e-02, 2.50000266e-02, 2.50019142e-02], [ 2.49947601e-02, 2.49989899e-02, 2.50019788e-02, ..., 2.50085934e-02, 2.49893379e-02, 2.49928418e-02]], [[-1.07791525e-05, -1.12762938e-05, -1.09048891e-05, ..., -3.03103984e-06, -6.97900310e-06, -9.55405245e-06], [-1.31395421e-05, -1.19425737e-05, -1.09475818e-05, ..., -6.44635932e-06, -1.04541963e-05, -1.29534313e-05], [-1.27915349e-05, -1.11171401e-05, -9.76481528e-06, ..., -9.51691022e-06, -1.21331660e-05, -1.35229631e-05], ... [ 2.60248830e-03, 7.61558494e-03, 1.65967402e-02, ..., 1.40826089e-02, 2.87408526e-03, -4.29198768e-04], [ 1.22832259e-02, 4.29871125e-02, 7.37550382e-02, ..., 1.46928229e-02, -6.55289911e-03, -7.48834501e-03], [ 4.38557079e-02, 7.57073251e-02, 9.79083413e-02, ..., 2.29154142e-02, 7.17551287e-03, 1.39742722e-02]], [[-2.69217144e-03, 2.86237508e-03, 8.24855009e-03, ..., -6.52199637e-03, -7.81110359e-03, -6.59392472e-03], [ 1.64599549e-03, 6.61193633e-03, 9.76250040e-03, ..., -9.89275327e-03, -8.16499031e-03, -3.86577442e-03], [ 3.17502149e-03, 4.99373977e-03, 5.28557787e-03, ..., -8.36652542e-03, -4.57271920e-03, -2.80895870e-04], ..., [-3.89758561e-03, -4.63884114e-03, -4.63652124e-03, ..., -1.48931238e-03, -2.48091221e-03, -3.13928397e-03], [-3.40594151e-03, -1.25105065e-03, 1.54065687e-03, ..., -2.23361267e-03, -4.23543444e-03, -4.57318246e-03], [-4.12094602e-03, 3.14988155e-05, 4.85938486e-03, ..., -3.67632372e-03, -6.24166405e-03, -6.47178273e-03]]]])
- uh(time, lev, l, k)complex128(-0.012658444856716517-0.0105831...
- long_name :
- zonal velocity anomaly in spectral space
- units :
- m s^-1
array([[[[-1.26584449e-02-1.05831945e-02j, -2.63625473e-02+1.66437369e-02j, -3.25108303e-02+3.32015791e-03j, ..., -2.25306696e-05-4.26595961e-02j, 1.91932096e-02-2.81772765e-02j, -1.03762516e-03-2.23862927e-05j], [-6.46876770e-03-8.42492000e-03j, -4.40062009e-02+8.94681726e-03j, -3.04430038e-02-3.62080810e-03j, ..., -5.25943744e-03-3.77062407e-02j, 8.61252339e-03-4.40776744e-02j, 9.78076148e-04+2.23862927e-05j], [-3.40017186e-02-3.50678122e-02j, -1.03328488e-02-1.42481275e-02j, -1.33130264e-02+2.45508712e-02j, ..., 3.06980282e-02-1.41362532e-02j, -1.63163628e-02-1.05300607e-02j, 5.33046816e-04-2.23862927e-05j], ..., [-4.38194988e-02-4.37546122e-02j, ... -1.58402232e-03+1.04014773e-04j], ..., [ 1.30796027e+01+1.30886761e+01j, 5.75503942e+00-1.11890465e+01j, -8.03963026e+00+2.97371180e+00j, ..., 3.63799773e+00-9.80735927e+00j, -1.23477102e+01+6.33513914e+00j, -4.53668993e-03-1.04014773e-04j], [ 2.73152274e+01+2.73036835e+01j, 1.01325183e+01-1.77162343e+01j, -5.36777816e+00-4.12989214e+00j, ..., -5.04455482e+00-6.52111086e+00j, -1.95564132e+01+1.11853285e+01j, 5.77195851e-03+1.04014773e-04j], [ 2.95620949e+01+2.95361891e+01j, 1.17262730e+01-2.08155471e+01j, 2.81507707e+00-7.87004397e+00j, ..., -9.63011385e+00+3.43643806e+00j, -2.29558497e+01+1.29410945e+01j, 1.29529103e-02-1.04014773e-04j]]]])
- uq_subgrid_flux(time, lev, y, x)float645.839e-13 3.404e-13 ... -1.171e-10
array([[[[ 5.83934752e-13, 3.40430975e-13, -2.81376359e-13, ..., 4.04675114e-13, 1.21691451e-12, -4.66429074e-13], [ 9.57383667e-13, -6.25629228e-13, -6.89132320e-13, ..., -1.24286342e-12, 1.64585402e-12, -9.09261093e-13], [ 9.18377186e-13, -1.28860482e-13, 7.56288183e-13, ..., -1.00824404e-12, -1.31349048e-13, -2.55796539e-13], ..., [-6.16000676e-13, 6.54135561e-14, 5.31346480e-13, ..., 1.29064785e-12, -8.26568869e-13, -6.12379447e-13], [-7.02366133e-13, -9.98798922e-14, 6.38842222e-13, ..., -1.16907233e-12, -1.32998039e-13, -5.18210441e-13], [ 6.81759951e-13, 6.33306256e-13, 5.23084215e-13, ..., -2.85367950e-12, 1.47111343e-12, 1.57800357e-13]], [[ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], ... [-6.08603078e-09, -1.96580321e-08, -5.78229971e-08, ..., -1.40096522e-09, -1.01426278e-08, -1.71088484e-09], [-4.82310801e-08, 5.85573535e-10, 1.06059372e-07, ..., 2.06393287e-08, 5.18209735e-09, -2.78377778e-08], [ 3.82569014e-08, 6.18158707e-08, 2.36361431e-08, ..., 2.27943426e-08, -2.20327886e-08, -4.62761363e-08]], [[-1.71875909e-09, -2.01290039e-09, -1.43090055e-09, ..., -4.22520828e-10, 1.69992867e-11, -7.43301185e-10], [-8.08192554e-10, -6.16857979e-10, -1.05430796e-10, ..., -5.03042958e-11, -5.78085383e-10, -1.03312375e-09], [-2.79976104e-10, 1.66831037e-10, 4.98960136e-10, ..., -1.55770771e-10, 2.52789821e-10, 2.13499522e-10], ..., [ 7.81286739e-11, 1.23305689e-10, -2.58996372e-10, ..., -1.24303099e-10, -1.21587386e-10, -8.04525697e-11], [-6.37064616e-12, -2.96563600e-10, -9.34455477e-10, ..., -4.32982133e-10, -3.49367027e-10, -2.26709109e-10], [-4.47652447e-10, -1.15226393e-09, -1.53668154e-09, ..., -5.26729774e-10, -1.62588266e-10, -1.17077443e-10]]]])
- uu_subgrid_flux(time, lev, y, x)float643.863e-10 1.245e-09 ... 1.612e-06
array([[[[ 3.86317333e-10, 1.24465245e-09, 2.29942580e-09, ..., -6.09837947e-10, 2.75967344e-10, -1.14583803e-09], [ 3.03318487e-09, 5.78759634e-09, 5.36699482e-09, ..., 3.76983496e-09, 2.61940161e-09, 3.25776814e-09], [ 6.41061205e-10, -7.69201345e-10, 1.67797024e-09, ..., -6.22014370e-10, 1.62277878e-10, -5.26771379e-10], ..., [ 4.79402064e-09, 6.27316960e-09, 4.59790265e-09, ..., 3.36808696e-09, 4.33761993e-09, 6.77195912e-09], [ 5.23460434e-10, -3.74432067e-10, 5.91982813e-10, ..., -4.20375218e-10, 8.75035261e-10, -1.27510981e-10], [ 2.93160986e-09, 4.47226287e-09, 3.37748132e-09, ..., 3.64276495e-09, 2.69066144e-09, 3.78701531e-09]], [[ 6.97363770e-12, 7.01413472e-12, 6.52648727e-12, ..., 8.85058398e-12, 8.57312606e-12, 7.08338830e-12], [ 2.83091184e-12, 4.26947624e-12, 3.97145292e-12, ..., 9.15564334e-12, 7.89431187e-12, 4.28753517e-12], [ 4.83864644e-13, 6.08313734e-13, 7.83352498e-13, ..., 6.13784330e-12, 2.52765467e-12, 1.23244063e-13], ... [ 1.20484863e-04, 4.94376957e-04, 1.06389001e-03, ..., 8.06687487e-05, 6.28816164e-05, 4.78843532e-05], [ 5.05621890e-04, 1.05710990e-03, 1.24275806e-03, ..., 2.55895121e-04, 1.81958079e-04, 1.92979013e-04], [ 1.07981433e-03, 6.66777717e-04, 3.34221103e-04, ..., 2.18614991e-04, 4.73086655e-04, 1.00958035e-03]], [[ 1.35406536e-05, 1.88921948e-05, 1.44045368e-05, ..., 6.95643951e-06, 2.42412610e-06, 4.58433188e-06], [ 1.78837941e-05, 1.05291918e-05, 5.31069266e-06, ..., 4.90037079e-06, 7.41882953e-06, 1.61909945e-05], [ 6.92769200e-06, 1.15289017e-05, 1.85113187e-05, ..., 1.38773206e-05, 1.50164312e-05, 9.69632424e-06], ..., [ 2.46370545e-06, 6.81261368e-06, 1.48766773e-05, ..., 8.90946619e-07, 4.19159985e-07, 5.66967627e-07], [ 1.92707256e-06, 5.45651909e-06, 1.28864539e-05, ..., 2.48778144e-06, 3.04364261e-06, 2.68794027e-06], [ 4.85063114e-06, 8.48369458e-06, 9.55611402e-06, ..., 5.27648466e-06, 2.02521318e-06, 1.61237113e-06]]]])
- uv_subgrid_flux(time, lev, y, x)float641.728e-07 -1.705e-07 ... -8.823e-07
array([[[[ 1.72835134e-07, -1.70482543e-07, 1.76164058e-07, ..., -1.83125656e-07, 1.69903862e-07, -1.71026294e-07], [ 1.67525394e-07, -1.67931012e-07, 1.67011324e-07, ..., -1.79491540e-07, 1.67299645e-07, -1.72353873e-07], [ 1.83633692e-07, -1.83914759e-07, 1.85005047e-07, ..., -1.84172918e-07, 1.86849162e-07, -1.88732854e-07], ..., [ 1.74507071e-07, -1.73637226e-07, 1.77444516e-07, ..., -1.74504041e-07, 1.77660939e-07, -1.74244596e-07], [ 1.69824899e-07, -1.72613351e-07, 1.68413050e-07, ..., -1.63913543e-07, 1.72695327e-07, -1.70805725e-07], [ 1.69558466e-07, -1.72821362e-07, 1.70029442e-07, ..., -1.83583007e-07, 1.68240476e-07, -1.69534567e-07]], [[ 2.77251441e-11, 5.46114611e-12, 3.85439656e-12, ..., -1.20026515e-11, -9.54238172e-12, 1.87632395e-11], [-3.34313687e-11, -2.98603813e-11, -3.43152161e-11, ..., -1.41163356e-11, -2.60443922e-11, 9.31630832e-12], [-3.36462438e-11, -2.66688596e-11, -1.74698693e-11, ..., 2.29734262e-12, -5.68895705e-12, 7.11698507e-12], ... [-3.06042268e-05, -6.52116758e-05, -3.00728633e-04, ..., -5.08456315e-05, -5.50698008e-05, -3.14113423e-05], [-3.82664172e-04, -6.91538718e-04, -6.86232566e-04, ..., -2.25054502e-06, 4.09687603e-05, -3.43677158e-05], [-8.05193399e-04, -4.64129024e-04, -1.68657531e-04, ..., 1.09723225e-05, 7.88947931e-05, -5.14666526e-04]], [[-1.44150233e-05, -1.59164875e-05, -8.28513989e-06, ..., -4.84033142e-06, -1.12599435e-06, -5.91181128e-06], [-1.88720081e-05, -8.28857633e-06, 9.71139069e-08, ..., -2.53374626e-06, -9.23871350e-06, -1.95729322e-05], [-4.47886951e-06, -4.47727922e-07, -1.26506081e-06, ..., -1.02282053e-05, -1.64932634e-05, -1.28313013e-05], ..., [ 1.05067472e-06, 9.65730632e-07, -1.43207737e-06, ..., -1.52248686e-06, -1.35899739e-06, -3.33126613e-07], [-5.63652955e-07, -3.80127847e-06, -6.83465757e-06, ..., -3.41695452e-06, -1.96415925e-06, 6.55649561e-07], [-3.85208213e-06, -7.03858754e-06, -7.21939009e-06, ..., -4.98378723e-06, -1.68604989e-06, -8.82271719e-07]]]])
- v(time, lev, y, x)float64-0.001415 -0.001645 ... 0.006776
- long_name :
- meridional velocity anomaly
- units :
- m s^-1
array([[[[-1.41478031e-03, -1.64527395e-03, -1.85446328e-03, ..., -1.82829320e-03, -1.23621537e-03, -1.29736961e-03], [-1.41177135e-03, -1.64307544e-03, -1.85863773e-03, ..., -1.80977921e-03, -1.21642953e-03, -1.29247943e-03], [-1.43826334e-03, -1.65736120e-03, -1.85511408e-03, ..., -1.78807862e-03, -1.19897675e-03, -1.29215385e-03], ..., [-1.42097237e-03, -1.64463736e-03, -1.85362004e-03, ..., -1.87232771e-03, -1.24113205e-03, -1.28212590e-03], [-1.41888970e-03, -1.64449518e-03, -1.85074687e-03, ..., -1.88265648e-03, -1.24646955e-03, -1.29415410e-03], [-1.42246682e-03, -1.64619221e-03, -1.84632595e-03, ..., -1.85878804e-03, -1.24681950e-03, -1.29746363e-03]], [[-1.51863921e-03, -1.59248245e-03, -1.65597169e-03, ..., -1.46232969e-03, -1.44765011e-03, -1.46377173e-03], [-1.51848253e-03, -1.59297075e-03, -1.65688749e-03, ..., -1.45839115e-03, -1.44398087e-03, -1.46211970e-03], [-1.51990903e-03, -1.59455991e-03, -1.65787548e-03, ..., -1.45496206e-03, -1.44094292e-03, -1.46132792e-03], ... [ 4.42463655e-02, 2.74781343e-02, 7.21897361e-03, ..., 3.47955417e-03, 1.60503861e-02, 4.07269957e-02], [ 2.89895730e-02, 7.45590950e-03, -1.42713492e-02, ..., 2.24673881e-02, 2.63844510e-02, 3.48271647e-02], [-4.13237161e-03, -2.62935629e-02, -3.62328918e-02, ..., 4.75445357e-02, 3.89193854e-02, 1.80472697e-02]], [[ 3.61383626e-04, -4.03762117e-03, -6.25296882e-03, ..., 8.47644018e-03, 8.24807077e-03, 5.06373449e-03], [-5.14913731e-03, -9.29200992e-03, -9.86033400e-03, ..., 9.59362685e-03, 6.80255011e-03, 1.05608327e-03], [-9.49466115e-03, -1.18198364e-02, -1.06885344e-02, ..., 7.75142077e-03, 2.67655770e-03, -3.98064834e-03], ..., [ 7.58692913e-03, 6.12077804e-03, 4.15312459e-03, ..., 8.18613123e-04, 4.73741923e-03, 7.62707783e-03], [ 7.17023724e-03, 5.09135174e-03, 2.51627651e-03, ..., 2.60101989e-03, 5.67111824e-03, 7.64362989e-03], [ 4.52251767e-03, 1.33655144e-03, -1.33073326e-03, ..., 5.60114106e-03, 7.19727286e-03, 6.77550659e-03]]]])
- v_subgrid_forcing(time, lev, y, x)float642.498e-13 1.586e-12 ... 8.655e-11
array([[[[ 2.49814210e-13, 1.58619399e-12, -1.59284904e-12, ..., 2.22197230e-12, 4.07754295e-13, 1.14557781e-13], [ 1.21016479e-12, -1.37559299e-12, -6.85925759e-13, ..., -8.76150101e-14, 1.24400409e-12, -9.43430799e-13], [ 1.24770068e-12, 4.83063053e-14, 1.41277014e-12, ..., -1.35644168e-12, -1.00716686e-12, 1.97503724e-13], ..., [-1.05124977e-12, 2.62542520e-13, 4.11850707e-13, ..., 5.81359132e-13, -7.47119680e-13, -1.29693037e-12], [-1.20043506e-12, -4.55040819e-13, 1.49779842e-12, ..., -1.49522371e-12, -1.78073808e-13, -9.95194549e-13], [ 1.92475080e-12, 6.77965502e-13, 1.42531873e-12, ..., -2.80216778e-12, 1.73423444e-12, 3.45626607e-14]], [[ 4.14002900e-16, 3.40146740e-15, -4.63591673e-16, ..., -4.67123775e-15, 1.94437300e-15, 3.73647544e-15], [ 6.23308629e-15, -5.80142509e-15, 6.44156436e-15, ..., -7.80205614e-15, 5.33191179e-15, 2.75873749e-16], [ 1.98271037e-15, -1.17565748e-15, 1.09978568e-15, ..., -4.21385073e-15, 2.97222301e-15, 1.74603845e-15], ... [ 5.84193375e-09, 9.81745412e-09, 8.34135785e-10, ..., -7.50942025e-10, 1.25705321e-09, -2.29696204e-09], [ 9.01672434e-09, -3.92406370e-09, 1.45487319e-08, ..., 9.47727884e-09, 3.14810729e-10, -1.86937537e-08], [ 2.27287436e-09, -5.81750222e-10, -2.15722740e-09, ..., 4.96600559e-10, -1.29711070e-09, -2.20746471e-08]], [[ 9.41792113e-11, 4.48263127e-10, 1.93133442e-10, ..., 3.32646405e-10, 1.41864486e-10, 4.46285880e-10], [ 1.25823040e-10, 1.10223889e-10, -1.51871954e-10, ..., 1.35329750e-10, 9.98355546e-11, 1.89115044e-10], [-4.05853718e-10, -1.19598389e-10, 5.91801883e-11, ..., -1.93623461e-10, -2.14782287e-10, -2.33763487e-10], ..., [ 7.49732506e-11, -8.55985311e-11, -2.59787367e-11, ..., -7.61304078e-11, -3.73784976e-12, 2.57973226e-11], [-5.84160047e-12, 1.49366348e-10, 1.29515681e-10, ..., 8.58276368e-11, 5.52669487e-11, 6.61769726e-11], [ 2.26478787e-10, 3.01806250e-10, 2.47331094e-10, ..., 9.97075601e-11, 1.03560942e-10, 8.65480665e-11]]]])
- vfull(time, lev, y, x)float64-0.001415 -0.001645 ... 0.006776
- long_name :
- meridional full velocities in real space
- units :
- m s^-1
array([[[[-1.41478031e-03, -1.64527395e-03, -1.85446328e-03, ..., -1.82829320e-03, -1.23621537e-03, -1.29736961e-03], [-1.41177135e-03, -1.64307544e-03, -1.85863773e-03, ..., -1.80977921e-03, -1.21642953e-03, -1.29247943e-03], [-1.43826334e-03, -1.65736120e-03, -1.85511408e-03, ..., -1.78807862e-03, -1.19897675e-03, -1.29215385e-03], ..., [-1.42097237e-03, -1.64463736e-03, -1.85362004e-03, ..., -1.87232771e-03, -1.24113205e-03, -1.28212590e-03], [-1.41888970e-03, -1.64449518e-03, -1.85074687e-03, ..., -1.88265648e-03, -1.24646955e-03, -1.29415410e-03], [-1.42246682e-03, -1.64619221e-03, -1.84632595e-03, ..., -1.85878804e-03, -1.24681950e-03, -1.29746363e-03]], [[-1.51863921e-03, -1.59248245e-03, -1.65597169e-03, ..., -1.46232969e-03, -1.44765011e-03, -1.46377173e-03], [-1.51848253e-03, -1.59297075e-03, -1.65688749e-03, ..., -1.45839115e-03, -1.44398087e-03, -1.46211970e-03], [-1.51990903e-03, -1.59455991e-03, -1.65787548e-03, ..., -1.45496206e-03, -1.44094292e-03, -1.46132792e-03], ... [ 4.42463655e-02, 2.74781343e-02, 7.21897361e-03, ..., 3.47955417e-03, 1.60503861e-02, 4.07269957e-02], [ 2.89895730e-02, 7.45590950e-03, -1.42713492e-02, ..., 2.24673881e-02, 2.63844510e-02, 3.48271647e-02], [-4.13237161e-03, -2.62935629e-02, -3.62328918e-02, ..., 4.75445357e-02, 3.89193854e-02, 1.80472697e-02]], [[ 3.61383626e-04, -4.03762117e-03, -6.25296882e-03, ..., 8.47644018e-03, 8.24807077e-03, 5.06373449e-03], [-5.14913731e-03, -9.29200992e-03, -9.86033400e-03, ..., 9.59362685e-03, 6.80255011e-03, 1.05608327e-03], [-9.49466115e-03, -1.18198364e-02, -1.06885344e-02, ..., 7.75142077e-03, 2.67655770e-03, -3.98064834e-03], ..., [ 7.58692913e-03, 6.12077804e-03, 4.15312459e-03, ..., 8.18613123e-04, 4.73741923e-03, 7.62707783e-03], [ 7.17023724e-03, 5.09135174e-03, 2.51627651e-03, ..., 2.60101989e-03, 5.67111824e-03, 7.64362989e-03], [ 4.52251767e-03, 1.33655144e-03, -1.33073326e-03, ..., 5.60114106e-03, 7.19727286e-03, 6.77550659e-03]]]])
- vh(time, lev, l, k)complex1280j ... 0.007440851489267999j
- long_name :
- meridional velocity anomaly in spectral space
- units :
- m s^-1
array([[[[ 0.00000000e+00+0.00000000e+00j, -1.56026941e+00-2.86581485e+00j, 1.77233862e-01-7.08276836e-01j, ..., -8.06243787e-01+4.90285025e-02j, -3.07104668e+00-1.91454672e+00j, 0.00000000e+00+9.28425534e-02j], [ 0.00000000e+00+0.00000000e+00j, -1.55845538e+00-2.86661970e+00j, 1.72653144e-01-6.97959315e-01j, ..., -8.00506820e-01+5.03366999e-02j, -3.06257697e+00-1.91321943e+00j, 0.00000000e+00+9.31606651e-02j], [ 0.00000000e+00+0.00000000e+00j, -1.56150538e+00-2.86885530e+00j, 1.74993128e-01-6.95458489e-01j, ..., -7.94385726e-01+5.31283433e-02j, -3.05524998e+00-1.91116473e+00j, 0.00000000e+00+8.97964891e-02j], ..., [ 0.00000000e+00+0.00000000e+00j, ... 0.00000000e+00-2.08073514e-02j], ..., [ 0.00000000e+00+0.00000000e+00j, 4.22483364e+00+2.06679116e+00j, 4.19210547e+00+4.66966415e+00j, ..., 5.65780070e+00+5.05028088e+00j, 2.18292839e+00+4.67068926e+00j, 0.00000000e+00+4.19297331e-02j], [ 0.00000000e+00+0.00000000e+00j, 2.77118373e+00+1.27498662e+00j, 4.07529287e+00+6.05828515e+00j, ..., 7.37135694e+00+4.88902572e+00j, 1.29784087e+00+3.08874180e+00j, 0.00000000e+00+4.18653633e-02j], [ 0.00000000e+00+0.00000000e+00j, 8.35852510e-01+1.60193985e-01j, 2.77083858e+00+6.36728817e+00j, ..., 7.79352204e+00+3.39368344e+00j, 9.08666140e-02+9.57669419e-01j, 0.00000000e+00+7.44085149e-03j]]]])
- vq_subgrid_flux(time, lev, y, x)float64-2.542e-12 1.62e-11 ... 1.934e-10
array([[[[-2.54209665e-12, 1.62027362e-11, -1.20084083e-11, ..., 8.17027403e-12, -1.57517908e-11, 2.35734342e-12], [-2.29508040e-12, 1.54580785e-11, -1.19851352e-11, ..., 8.67288645e-12, -1.60936817e-11, 2.17298397e-12], [-3.05743704e-12, 1.58338173e-11, -1.15755015e-11, ..., 1.11147675e-11, -1.55664576e-11, 2.92231949e-12], ..., [-3.84738640e-12, 1.63435247e-11, -1.10118298e-11, ..., 6.98933431e-12, -1.76739062e-11, 3.07457986e-12], [-3.00786436e-12, 1.67449812e-11, -1.07442327e-11, ..., 9.55379266e-12, -1.80189195e-11, 2.32530506e-12], [-3.31514295e-12, 1.66091113e-11, -1.19952484e-11, ..., 9.79456373e-12, -1.67115311e-11, 2.06195678e-12]], [[ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], ... [ 1.38602830e-08, 7.81550531e-09, 2.11142324e-08, ..., -8.86480396e-09, 1.27880313e-10, -2.93910809e-08], [ 5.47964178e-08, 1.46673429e-08, -5.55126731e-08, ..., -1.03648560e-08, -1.53975129e-08, 1.27335346e-08], [-3.49753832e-08, -8.65426017e-08, -3.75512792e-08, ..., 2.57841004e-09, 1.23813349e-08, 1.28747978e-08]], [[ 2.29133314e-09, 2.16838123e-09, 1.22497483e-09, ..., 4.19977204e-10, 2.51461788e-10, 1.34906309e-09], [ 7.21586171e-10, 4.04796057e-10, -1.77364759e-11, ..., 2.69587987e-10, 9.60520109e-10, 1.28594361e-09], [ 2.95300210e-10, 1.83241828e-10, -2.08666333e-10, ..., 2.38864579e-10, -2.15591163e-10, -4.04977170e-10], ..., [-3.21730801e-11, 3.15106682e-11, 1.65576980e-10, ..., 2.37049438e-10, 9.35931994e-11, 1.32606694e-10], [ 1.19620005e-11, 4.07894366e-10, 7.51480507e-10, ..., 5.88329189e-10, 3.91067733e-10, 7.47972184e-11], [ 1.05157653e-09, 1.87421026e-09, 1.84907765e-09, ..., 3.81266219e-10, 3.38015929e-11, 1.93401620e-10]]]])
- vv_subgrid_flux(time, lev, y, x)float643.388e-07 7.804e-07 ... 1.838e-06
array([[[[ 3.38820162e-07, 7.80365450e-07, 6.59708101e-07, ..., 1.42711155e-06, 5.52073353e-07, 4.46704058e-07], [ 3.47691496e-07, 7.74014296e-07, 6.46667281e-07, ..., 1.45055680e-06, 5.64957514e-07, 4.40581645e-07], [ 3.61607347e-07, 7.61222684e-07, 6.53462471e-07, ..., 1.42695480e-06, 5.59419020e-07, 4.34949687e-07], ..., [ 3.40299492e-07, 7.48044517e-07, 6.38278734e-07, ..., 1.46556619e-06, 5.54538945e-07, 4.61555589e-07], [ 3.16546733e-07, 7.50662795e-07, 6.51139097e-07, ..., 1.45811666e-06, 5.45511963e-07, 4.38510993e-07], [ 3.20033753e-07, 7.54654849e-07, 6.64496535e-07, ..., 1.42763201e-06, 5.54410958e-07, 4.42135180e-07]], [[ 6.61328094e-10, 3.12226114e-09, -5.23746289e-10, ..., 9.00134184e-10, -2.19285222e-10, 1.28319257e-09], [ 7.38256138e-10, 3.10623706e-09, -4.71939474e-10, ..., 8.60305875e-10, -1.98100665e-10, 1.30768390e-09], [ 8.01897804e-10, 3.06271016e-09, -4.74179021e-10, ..., 8.54196574e-10, -2.06370180e-10, 1.38323714e-09], ... [ 1.02070705e-04, 2.30970547e-04, 2.23477715e-04, ..., 1.27060843e-04, 3.15829416e-04, 2.47415413e-04], [ 3.78660936e-04, 5.55303323e-04, 4.86947831e-04, ..., 2.97175412e-04, 2.42466402e-04, 1.80134552e-04], [ 7.07505813e-04, 3.77549079e-04, 1.76026131e-04, ..., 2.52781767e-04, 3.23705915e-04, 3.78233086e-04]], [[ 1.68024862e-05, 1.62443213e-05, 8.91000492e-06, ..., 3.79127252e-06, 2.91062144e-06, 9.69709341e-06], [ 2.09582380e-05, 1.00482516e-05, 2.38188335e-06, ..., 3.31780303e-06, 1.22436156e-05, 2.38849792e-05], [ 8.88515700e-06, 2.97456375e-06, 1.55490442e-06, ..., 8.94610455e-06, 2.02004968e-05, 2.11088030e-05], ..., [ 9.88897722e-07, 1.36997176e-06, 2.11995358e-06, ..., 4.75970886e-06, 5.37242488e-06, 1.56008490e-06], [ 2.50996702e-06, 4.78743745e-06, 5.79682207e-06, ..., 5.29605177e-06, 3.87123124e-06, 1.20909090e-06], [ 7.62194946e-06, 1.06231050e-05, 8.78019732e-06, ..., 6.08793854e-06, 2.10727380e-06, 1.83784368e-06]]]])
- kPandasIndex
PandasIndex(Float64Index([ 0.0, 6.283185307179587e-06, 1.2566370614359173e-05, 1.8849555921538758e-05, 2.5132741228718347e-05, 3.1415926535897935e-05, 3.7699111843077517e-05, 4.3982297150257105e-05, 5.026548245743669e-05, 5.654866776461628e-05, 6.283185307179587e-05, 6.911503837897546e-05, 7.539822368615503e-05, 8.168140899333462e-05, 8.796459430051421e-05, 9.42477796076938e-05, 0.00010053096491487339, 0.00010681415022205297, 0.00011309733552923256, 0.00011938052083641215, 0.00012566370614359174, 0.00013194689145077133, 0.00013823007675795092, 0.0001445132620651305, 0.00015079644737231007, 0.00015707963267948965, 0.00016336281798666924, 0.00016964600329384883, 0.00017592918860102842, 0.000182212373908208, 0.0001884955592153876, 0.00019477874452256718, 0.00020106192982974677], dtype='float64', name='k'))
- lPandasIndex
PandasIndex(Float64Index([ 0.0, 6.283185307179587e-06, 1.2566370614359173e-05, 1.8849555921538758e-05, 2.5132741228718347e-05, 3.1415926535897935e-05, 3.7699111843077517e-05, 4.3982297150257105e-05, 5.026548245743669e-05, 5.654866776461628e-05, 6.283185307179587e-05, 6.911503837897546e-05, 7.539822368615503e-05, 8.168140899333462e-05, 8.796459430051421e-05, 9.42477796076938e-05, 0.00010053096491487339, 0.00010681415022205297, 0.00011309733552923256, 0.00011938052083641215, 0.00012566370614359174, 0.00013194689145077133, 0.00013823007675795092, 0.0001445132620651305, 0.00015079644737231007, 0.00015707963267948965, 0.00016336281798666924, 0.00016964600329384883, 0.00017592918860102842, 0.000182212373908208, 0.0001884955592153876, 0.00019477874452256718, -0.00020106192982974677, -0.00019477874452256718, -0.0001884955592153876, -0.000182212373908208, -0.00017592918860102842, -0.00016964600329384883, -0.00016336281798666924, -0.00015707963267948965, -0.00015079644737231007, -0.0001445132620651305, -0.00013823007675795092, -0.00013194689145077133, -0.00012566370614359174, -0.00011938052083641215, -0.00011309733552923256, -0.00010681415022205297, -0.00010053096491487339, -9.42477796076938e-05, -8.796459430051421e-05, -8.168140899333462e-05, -7.539822368615503e-05, -6.911503837897546e-05, -6.283185307179587e-05, -5.654866776461628e-05, -5.026548245743669e-05, -4.3982297150257105e-05, -3.7699111843077517e-05, -3.1415926535897935e-05, -2.5132741228718347e-05, -1.8849555921538758e-05, -1.2566370614359173e-05, -6.283185307179587e-06], dtype='float64', name='l'))
- levPandasIndex
PandasIndex(Int64Index([1, 2], dtype='int64', name='lev'))
- lev_midPandasIndex
PandasIndex(Float64Index([1.5], dtype='float64', name='lev_mid'))
- timePandasIndex
PandasIndex(Float64Index([0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0], dtype='float64', name='time'))
- xPandasIndex
PandasIndex(Float64Index([ 7812.5, 23437.5, 39062.5, 54687.5, 70312.5, 85937.5, 101562.5, 117187.5, 132812.5, 148437.5, 164062.5, 179687.5, 195312.5, 210937.5, 226562.5, 242187.5, 257812.5, 273437.5, 289062.5, 304687.5, 320312.5, 335937.5, 351562.5, 367187.5, 382812.5, 398437.5, 414062.5, 429687.5, 445312.5, 460937.5, 476562.5, 492187.5, 507812.5, 523437.5, 539062.5, 554687.5, 570312.5, 585937.5, 601562.5, 617187.5, 632812.5, 648437.5, 664062.5, 679687.5, 695312.5, 710937.5, 726562.5, 742187.5, 757812.5, 773437.5, 789062.5, 804687.5, 820312.5, 835937.5, 851562.5, 867187.5, 882812.5, 898437.5, 914062.5, 929687.5, 945312.5, 960937.5, 976562.5, 992187.5], dtype='float64', name='x'))
- yPandasIndex
PandasIndex(Float64Index([ 7812.5, 23437.5, 39062.5, 54687.5, 70312.5, 85937.5, 101562.5, 117187.5, 132812.5, 148437.5, 164062.5, 179687.5, 195312.5, 210937.5, 226562.5, 242187.5, 257812.5, 273437.5, 289062.5, 304687.5, 320312.5, 335937.5, 351562.5, 367187.5, 382812.5, 398437.5, 414062.5, 429687.5, 445312.5, 460937.5, 476562.5, 492187.5, 507812.5, 523437.5, 539062.5, 554687.5, 570312.5, 585937.5, 601562.5, 617187.5, 632812.5, 648437.5, 664062.5, 679687.5, 695312.5, 710937.5, 726562.5, 742187.5, 757812.5, 773437.5, 789062.5, 804687.5, 820312.5, 835937.5, 851562.5, 867187.5, 882812.5, 898437.5, 914062.5, 929687.5, 945312.5, 960937.5, 976562.5, 992187.5], dtype='float64', name='y'))
- pyqg:L :
- 1000000.0
- pyqg:M :
- 4096
- pyqg:W :
- 1000000.0
- pyqg:beta :
- 1.5e-11
- pyqg:del2 :
- 0.8
- pyqg:delta :
- 0.25
- pyqg:dt :
- 3600.0
- pyqg:filterfac :
- 23.6
- pyqg:nk :
- 33
- pyqg:nl :
- 64
- pyqg:ntd :
- 1
- pyqg:nx :
- 64
- pyqg:ny :
- 64
- pyqg:nz :
- 2
- pyqg:rd :
- 15000.0
- pyqg:rek :
- 5.787e-07
- pyqg:taveint :
- 86400.0
- pyqg:tavestart :
- 315360000.0
- pyqg:tc :
- 0
- pyqg:tmax :
- 1576800000.0
- pyqg:twrite :
- 1000.0
- reference :
- https://pyqg.readthedocs.io/en/latest/index.html
- title :
- pyqg: Python Quasigeostrophic Model
The parameterization we are using for this example is an FCNN trained on the values of PV and velocity to estimate PV subgrid forcing computed with Operator 2.
param = FCNNParameterization.train_on(training_ds, '/home/jovyan/models/fcnn_qu_to_Sq2',
inputs=['q', 'u', 'v'],
targets = ['q_subgrid_forcing'])
Now that we have a trained parameterization we can begin testing it. We call test_offline()
on the parameterization instance to begin evaluating the parameterization on an offline dataset.
# Helpers methods to help visualize offline metrics/performance
def imshow(arr):
plt.imshow(arr, vmin=0, vmax=1, cmap='inferno')
mean = arr.mean().data
plt.text(32, 32, f"{mean:.2f}", color=('white' if mean<0.75 else 'black'),
fontweight='bold', ha='center', va='center', fontsize=16)
plt.xticks([]); plt.yticks([])
def colorbar(label):
plt.colorbar().set_label(label, fontsize=16,rotation=0,ha='left',va='center')
Upon calling test_offline()
, an xarray.Dataset
object describing the predictions made by the parameterization is returned. Included within this dataset are a number of computed metrics.
test_ds = xr.open_zarr('/home/jovyan/datasets/eddy/op2/ds2').load()
preds1 = param.test_offline(test_ds)
preds1
<pyqg_parameterization_benchmarks.neural_networks.FCNNParameterization object at 0x7f70e74a8d30>
<xarray.Dataset> Dimensions: (time: 87, lev: 2, y: 64, x: 64) Coordinates: * lev (lev) int64 1 2 * time (time) float64 0.0 0.0 ... 0.0 0.0 * x (x) float64 7.812e+03 ... 9.922e+05 * y (y) float64 7.812e+03 ... 9.922e+05 Data variables: (12/14) q_subgrid_forcing (time, lev, y, x) float64 5.552e-... q_subgrid_forcing_predictions (time, lev, y, x) float64 -6.531e... q_subgrid_forcing_spatial_mse (lev, y, x) float64 1.242e-23 ...... q_subgrid_forcing_temporal_mse (time, lev) float64 2.242e-27 ...... q_subgrid_forcing_mse (lev) float64 9.821e-24 1.093e-27 q_subgrid_forcing_spatial_skill (lev, y, x) float64 0.0204 ... 0.... ... ... q_subgrid_forcing_spatial_correlation (lev, y, x) float64 0.1719 ... 0.... q_subgrid_forcing_temporal_correlation (time, lev) float64 0.004937 ... ... q_subgrid_forcing_correlation (lev) float64 0.5452 0.8939 correlation (lev) float64 0.5452 0.8939 mse (lev) float64 9.821e-24 1.093e-27 skill (lev) float64 0.2967 0.7989 Attributes: (12/23) pyqg:L: 1000000.0 pyqg:M: 4096 pyqg:W: 1000000.0 pyqg:beta: 1.5e-11 pyqg:del2: 0.8 pyqg:delta: 0.25 ... ... pyqg:tavestart: 315360000.0 pyqg:tc: 0 pyqg:tmax: 1576800000.0 pyqg:twrite: 1000.0 reference: https://pyqg.readthedocs.io/en/latest/index.html title: pyqg: Python Quasigeostrophic Model
- time: 87
- lev: 2
- y: 64
- x: 64
- lev(lev)int641 2
- long_name :
- vertical levels
array([1, 2])
- time(time)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- long_name :
- model time
- units :
- s
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
- x(x)float647.812e+03 2.344e+04 ... 9.922e+05
- long_name :
- real space grid points in the x direction
- units :
- grid point
array([ 7812.5, 23437.5, 39062.5, 54687.5, 70312.5, 85937.5, 101562.5, 117187.5, 132812.5, 148437.5, 164062.5, 179687.5, 195312.5, 210937.5, 226562.5, 242187.5, 257812.5, 273437.5, 289062.5, 304687.5, 320312.5, 335937.5, 351562.5, 367187.5, 382812.5, 398437.5, 414062.5, 429687.5, 445312.5, 460937.5, 476562.5, 492187.5, 507812.5, 523437.5, 539062.5, 554687.5, 570312.5, 585937.5, 601562.5, 617187.5, 632812.5, 648437.5, 664062.5, 679687.5, 695312.5, 710937.5, 726562.5, 742187.5, 757812.5, 773437.5, 789062.5, 804687.5, 820312.5, 835937.5, 851562.5, 867187.5, 882812.5, 898437.5, 914062.5, 929687.5, 945312.5, 960937.5, 976562.5, 992187.5])
- y(y)float647.812e+03 2.344e+04 ... 9.922e+05
- long_name :
- real space grid points in the y direction
- units :
- grid point
array([ 7812.5, 23437.5, 39062.5, 54687.5, 70312.5, 85937.5, 101562.5, 117187.5, 132812.5, 148437.5, 164062.5, 179687.5, 195312.5, 210937.5, 226562.5, 242187.5, 257812.5, 273437.5, 289062.5, 304687.5, 320312.5, 335937.5, 351562.5, 367187.5, 382812.5, 398437.5, 414062.5, 429687.5, 445312.5, 460937.5, 476562.5, 492187.5, 507812.5, 523437.5, 539062.5, 554687.5, 570312.5, 585937.5, 601562.5, 617187.5, 632812.5, 648437.5, 664062.5, 679687.5, 695312.5, 710937.5, 726562.5, 742187.5, 757812.5, 773437.5, 789062.5, 804687.5, 820312.5, 835937.5, 851562.5, 867187.5, 882812.5, 898437.5, 914062.5, 929687.5, 945312.5, 960937.5, 976562.5, 992187.5])
- q_subgrid_forcing(time, lev, y, x)float645.552e-17 3.096e-16 ... -2.656e-14
array([[[[ 5.55179776e-17, 3.09560834e-16, -1.66063278e-16, ..., 5.13362079e-17, -1.20823996e-17, 4.36760085e-17], [ 6.95917707e-17, -2.92396179e-16, 2.26111701e-16, ..., 9.56804274e-17, 9.32421941e-17, -2.13893152e-16], [-5.62658759e-17, -2.31359958e-16, 1.52806560e-16, ..., -1.66016465e-16, 1.91860586e-16, -1.41295930e-16], ..., [ 1.09645570e-16, 1.06962694e-16, -4.35254919e-17, ..., -2.47803644e-16, 1.25878311e-16, -1.33889214e-16], [ 3.40285926e-17, 9.33771833e-17, -6.26269462e-17, ..., 7.91205656e-17, 1.39150640e-16, -2.52517433e-16], [-6.93781564e-17, 5.06652452e-17, 2.45310189e-17, ..., -1.31091093e-17, -1.69117439e-16, 2.75261631e-16]], [[ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], ... [-2.30867925e-11, -1.00058652e-11, 1.60266166e-12, ..., 1.06329297e-11, -1.67707475e-11, 5.95654608e-12], [ 2.53160477e-11, -2.71636402e-12, -2.98504797e-12, ..., 7.72276122e-14, 3.36663902e-12, -2.39130571e-11], [-2.41466025e-11, 1.51891312e-11, -1.63883657e-12, ..., -8.46028372e-12, 4.47933826e-13, 1.53905702e-11]], [[-1.45171667e-14, 5.74727108e-15, 8.18115069e-15, ..., 6.96897006e-15, 2.09970188e-14, 2.00784809e-14], [ 2.21630557e-14, 1.48563503e-14, 1.05098070e-15, ..., -2.64058250e-14, -4.40439393e-14, 9.04110287e-15], [ 6.62723099e-15, 1.59673282e-14, -1.70590056e-14, ..., -7.70482601e-14, -5.32238995e-14, 5.43393561e-14], ..., [-4.39536859e-14, -2.78042104e-14, -1.12086452e-13, ..., 1.88676590e-13, 5.59096073e-15, 3.58463440e-14], [-3.59522708e-14, -2.33749362e-14, 5.56709525e-15, ..., 9.79367839e-14, 1.72077204e-14, -5.29816496e-14], [ 1.10142620e-14, -3.08420529e-15, -1.41550306e-14, ..., -5.21726218e-14, -3.64078037e-14, -2.65578732e-14]]]])
- q_subgrid_forcing_predictions(time, lev, y, x)float64-6.531e-13 ... -8.378e-15
array([[[[-6.53083924e-13, -1.77470665e-14, -3.83374058e-15, ..., -4.08957163e-15, -1.15118503e-14, 1.46205734e-13], [ 7.83678203e-14, 3.17592844e-15, -2.15039558e-14, ..., 6.67962982e-15, 4.56255091e-16, -5.44672678e-14], [ 7.81388300e-14, 3.23079542e-15, -2.12602644e-14, ..., 6.60655460e-15, 3.99742482e-16, -5.45381577e-14], ..., [ 7.80373758e-14, 2.71465589e-15, -2.12373081e-14, ..., 7.11476335e-15, -5.93989464e-17, -5.46529646e-14], [ 7.81992811e-14, 3.08359295e-15, -2.13761487e-14, ..., 7.19018571e-15, 6.61157179e-16, -5.44762769e-14], [ 4.21370350e-13, 3.54059853e-13, 3.29913885e-13, ..., 3.52176459e-13, 3.50942501e-13, 3.30945937e-13]], [[-3.89340007e-15, -6.57220784e-15, -6.57159882e-15, ..., -6.42684301e-15, -6.41443736e-15, -4.29309628e-15], [ 8.53013953e-16, 3.49425231e-16, 3.30635235e-16, ..., 4.63696540e-16, 4.62275431e-16, -2.96169835e-16], [ 8.54493031e-16, 3.49690802e-16, 3.31496217e-16, ..., 4.65551331e-16, 4.64282475e-16, -2.96543403e-16], ... [-4.52085244e-12, -7.37308218e-13, 1.58558344e-12, ..., 1.81608764e-12, -8.46013190e-12, -5.86784365e-13], [ 3.57049264e-12, 1.10086799e-12, -2.32484258e-12, ..., -6.89357859e-12, -5.53686708e-13, -4.63843070e-12], [-2.47634053e-12, 8.70173345e-13, 1.62760539e-12, ..., -3.00290305e-12, 2.61340906e-12, 2.45534669e-12]], [[ 5.28521835e-15, -8.09020396e-15, 3.86329144e-15, ..., -1.16246937e-14, -3.74923125e-15, 1.22767380e-14], [ 1.00720501e-14, 8.42094660e-15, 1.10953625e-14, ..., -3.66820560e-14, -3.54049099e-14, 7.65673654e-15], [ 1.22452148e-14, 1.49732640e-14, -1.16354748e-14, ..., -5.17022507e-14, -5.68905546e-14, 1.02485396e-14], ..., [-2.93166472e-14, -3.48482365e-14, -1.00919334e-13, ..., 1.95562493e-13, 9.03601467e-14, 8.52090750e-15], [-2.09073346e-14, -1.36722457e-15, -5.68645269e-14, ..., 7.67898386e-14, -6.63476096e-14, -2.06464535e-14], [-7.52108982e-16, 1.30190033e-15, -4.47815943e-15, ..., -3.12957973e-14, -5.06248892e-14, -8.37791987e-15]]]])
- q_subgrid_forcing_spatial_mse(lev, y, x)float641.242e-23 1.46e-23 ... 1.413e-27
array([[[1.24229842e-23, 1.46030419e-23, 1.17950892e-23, ..., 1.44663290e-23, 8.47329804e-24, 1.15224519e-23], [1.20397105e-23, 7.68627926e-24, 8.92822995e-24, ..., 7.68686068e-24, 9.73481376e-24, 1.08720443e-23], [1.51492601e-23, 1.10574374e-23, 1.24187840e-23, ..., 5.18572153e-24, 9.95481868e-24, 1.47254277e-23], ..., [1.61702970e-23, 1.42190739e-23, 7.58177488e-24, ..., 9.20003919e-24, 7.15430474e-24, 1.82301696e-23], [1.44837834e-23, 9.45425221e-24, 8.06773464e-24, ..., 7.43818909e-24, 8.66679236e-24, 1.98459400e-23], [2.12213337e-23, 2.18271079e-23, 1.42929516e-23, ..., 8.02458847e-24, 1.09572255e-23, 1.86931915e-23]], [[1.37815964e-27, 1.39875176e-27, 1.11851416e-27, ..., 1.95334040e-27, 1.52906079e-27, 1.06809651e-27], [1.08241487e-27, 1.18717380e-27, 1.17896526e-27, ..., 6.84391010e-28, 1.02722354e-27, 1.13885962e-27], [1.20469164e-27, 7.88514038e-28, 1.12484535e-27, ..., 1.40488683e-27, 1.55880255e-27, 1.38112047e-27], ..., [1.16316296e-27, 9.26580931e-28, 1.11074351e-27, ..., 1.39557055e-27, 1.81713688e-27, 1.49734981e-27], [1.28864632e-27, 7.17684566e-28, 1.01814766e-27, ..., 1.40646401e-27, 1.18603708e-27, 1.64095055e-27], [2.25475505e-27, 1.59669599e-27, 1.56123031e-27, ..., 1.20294817e-27, 1.31266480e-27, 1.41347880e-27]]])
- q_subgrid_forcing_temporal_mse(time, lev)float642.242e-27 1.847e-30 ... 1.428e-27
array([[2.24194425e-27, 1.84736509e-30], [2.18361665e-27, 1.93900970e-30], [2.17645701e-27, 1.94266235e-30], [2.19420717e-27, 1.94137957e-30], [2.16228157e-27, 1.93603467e-30], [2.20671642e-27, 1.93047796e-30], [2.18480895e-27, 1.92114623e-30], [2.17047372e-27, 1.90585162e-30], [2.22956578e-27, 1.88438594e-30], [2.39148308e-27, 1.85133110e-30], [2.52167960e-27, 1.81600610e-30], [2.83633155e-27, 1.74375108e-30], [3.42321746e-27, 1.95638706e-30], [4.55001795e-27, 3.03675507e-30], [6.83765723e-27, 5.69148524e-30], [1.18766116e-26, 1.01910810e-29], [2.00682875e-26, 1.17167299e-29], [1.78019157e-26, 1.63363155e-29], [2.27053639e-26, 1.55565721e-29], [5.97894540e-26, 2.05459746e-29], ... [1.22003138e-23, 1.07927142e-27], [1.53755158e-23, 1.46181233e-27], [1.24756886e-23, 1.39791156e-27], [1.19842421e-23, 1.26512571e-27], [1.13963164e-23, 1.14361433e-27], [1.06456704e-23, 9.87429878e-28], [1.14205838e-23, 1.09977905e-27], [1.12090500e-23, 1.11767979e-27], [1.16208320e-23, 1.00319179e-27], [1.19699779e-23, 1.38183106e-27], [1.23269636e-23, 1.10528001e-27], [1.22370944e-23, 1.24924100e-27], [1.12006059e-23, 8.91332842e-28], [9.50334530e-24, 9.66912909e-28], [1.04441709e-23, 7.30895726e-28], [9.16805213e-24, 8.90772190e-28], [1.16708613e-23, 1.17113226e-27], [1.40098398e-23, 1.14184259e-27], [1.14759479e-23, 1.09752765e-27], [1.42664958e-23, 1.42774221e-27]])
- q_subgrid_forcing_mse(lev)float649.821e-24 1.093e-27
array([9.82143520e-24, 1.09267217e-27])
- q_subgrid_forcing_spatial_skill(lev, y, x)float640.0204 0.3126 ... 0.7333 0.5121
array([[[ 0.02040225, 0.31261365, 0.50300744, ..., 0.20818752, 0.29556292, 0.19168355], [ 0.3222494 , 0.21167634, 0.24327284, ..., 0.43993529, 0.40206677, 0.29467072], [-0.11781834, 0.20452293, 0.41856026, ..., 0.29634427, 0.03307484, 0.2702877 ], ..., [ 0.14179716, 0.39347524, 0.54122138, ..., 0.40227592, 0.60771686, 0.12419923], [ 0.31903549, 0.38716128, 0.10901203, ..., 0.38489406, 0.22492098, 0.21128348], [ 0.13675667, 0.25563562, 0.30881177, ..., 0.2514451 , 0.24265718, 0.23407802]], [[ 0.55517318, 0.65013044, 0.7688432 , ..., 0.71431715, 0.7398447 , 0.68679548], [ 0.76741682, 0.74225042, 0.75836764, ..., 0.8352333 , 0.75899464, 0.60014303], [ 0.71124943, 0.83311636, 0.82203912, ..., 0.89803532, 0.75941511, 0.71135351], ..., [ 0.78798429, 0.8320431 , 0.83478048, ..., 0.86021022, 0.85513528, 0.7601249 ], [ 0.69192014, 0.86639107, 0.81106474, ..., 0.87865351, 0.67658191, 0.60524401], [ 0.50467167, 0.74782752, 0.71624792, ..., 0.74588285, 0.73328292, 0.51213279]]])
- q_subgrid_forcing_temporal_skill(time, lev)float64-9.099e+04 -1.816e+30 ... 0.8189
array([[-9.09920498e+04, -1.81556992e+30], [-1.00614273e+05, -4.82420521e+07], [-8.73479346e+04, -3.36277699e+07], [-8.13637101e+04, -2.27886804e+07], [-6.92110476e+04, -1.30910633e+07], [-5.32386440e+04, -6.45546572e+06], [-3.39780239e+04, -3.15686746e+06], [-2.55483864e+04, -1.66531390e+06], [-1.62557410e+04, -7.99600976e+05], [-1.07835570e+04, -4.14851536e+05], [-5.86734349e+03, -1.70009367e+05], [-3.41093468e+03, -6.40096888e+04], [-2.53781065e+03, -2.83211729e+04], [-1.73118171e+03, -1.86108774e+04], [-1.32643449e+03, -1.28709007e+04], [-1.17653595e+03, -8.55811299e+03], [-9.84163895e+02, -3.40204687e+03], [-3.27065140e+02, -1.47489431e+03], [-8.14034959e+01, -1.56438797e+02], [-2.87482094e+00, -2.08507703e+00], ... [ 2.97682969e-01, 8.11781966e-01], [ 2.84854275e-01, 8.09510645e-01], [ 3.61464477e-01, 7.79504220e-01], [ 2.72582342e-01, 7.97794407e-01], [ 2.95130837e-01, 8.17760783e-01], [ 2.91479541e-01, 8.15809039e-01], [ 3.10997460e-01, 8.18967516e-01], [ 3.41543200e-01, 8.02426663e-01], [ 2.95361142e-01, 8.27014526e-01], [ 3.41662187e-01, 7.85056702e-01], [ 3.00075420e-01, 8.11781261e-01], [ 3.07228911e-01, 7.81817403e-01], [ 2.70768854e-01, 8.30659270e-01], [ 3.05308453e-01, 8.03727817e-01], [ 3.14043584e-01, 7.96016911e-01], [ 2.82704026e-01, 8.31257902e-01], [ 3.05203480e-01, 7.87867403e-01], [ 3.05602889e-01, 8.19665732e-01], [ 2.41501095e-01, 8.07207144e-01], [ 2.76592154e-01, 8.18889124e-01]])
- q_subgrid_forcing_skill(lev)float640.2967 0.7989
array([0.29672359, 0.79886685])
- q_subgrid_forcing_spatial_correlation(lev, y, x)float640.1719 0.6038 ... 0.8637 0.7206
array([[[0.17187867, 0.60375873, 0.78559814, ..., 0.46868546, 0.55137374, 0.44043018], [0.60848806, 0.48485149, 0.52949764, ..., 0.65948407, 0.64575246, 0.57041376], [0.07628657, 0.47561797, 0.64994092, ..., 0.56033694, 0.30056952, 0.53022525], ..., [0.37037015, 0.65244586, 0.77445874, ..., 0.63529734, 0.798192 , 0.36124084], [0.65094822, 0.62847462, 0.3369839 , ..., 0.62597687, 0.47556083, 0.51388974], [0.39952 , 0.60597382, 0.59143129, ..., 0.48721956, 0.51272034, 0.61558032]], [[0.77976057, 0.80328863, 0.88402396, ..., 0.90832107, 0.87661871, 0.86287841], [0.89126457, 0.86600992, 0.87175007, ..., 0.92142015, 0.87232597, 0.77703854], [0.85647501, 0.91479325, 0.90694552, ..., 0.9544756 , 0.87270492, 0.85856382], ..., [0.9093613 , 0.9122271 , 0.91870262, ..., 0.92765291, 0.94245784, 0.90998051], [0.85923528, 0.93317306, 0.90160708, ..., 0.94771885, 0.84097037, 0.80034969], [0.78578065, 0.87910578, 0.8571766 , ..., 0.86783168, 0.86370817, 0.72055781]]])
- q_subgrid_forcing_temporal_correlation(time, lev)float640.004937 nan ... 0.526 0.9049
array([[ 4.93699290e-03, nan], [ 7.19499051e-03, -4.24970673e-02], [-2.08940052e-03, -2.72713942e-02], [-4.98570878e-03, -1.94131225e-02], [-2.06616397e-02, -3.75083571e-03], [-2.42763270e-03, 9.08439359e-03], [-3.33035461e-03, 2.28887090e-02], [-9.13355408e-03, -3.03087896e-02], [-9.73282987e-03, -9.63916659e-02], [-8.36360564e-03, -8.14199227e-02], [ 8.25615977e-04, -5.02774396e-02], [-1.29883347e-02, -6.83216817e-02], [ 6.58153013e-03, -8.35406766e-02], [-5.83242760e-04, -5.47126782e-02], [ 8.71646491e-04, -2.80322415e-02], [-1.89189597e-03, -2.00602567e-02], [-1.21319586e-03, -2.44015341e-03], [-8.39823334e-04, 1.23577484e-02], [ 2.17433361e-02, 4.68852300e-02], [ 1.35627875e-01, 4.09010997e-01], ... [ 5.45606119e-01, 9.01064674e-01], [ 5.36676802e-01, 8.99739099e-01], [ 6.03263886e-01, 8.83903117e-01], [ 5.22321301e-01, 8.93195513e-01], [ 5.45396744e-01, 9.04328350e-01], [ 5.39960048e-01, 9.03288949e-01], [ 5.58299249e-01, 9.05051168e-01], [ 5.84817866e-01, 8.95786745e-01], [ 5.45336371e-01, 9.09574914e-01], [ 5.87126374e-01, 8.86281069e-01], [ 5.47812108e-01, 9.00989784e-01], [ 5.57685756e-01, 8.85201230e-01], [ 5.21182996e-01, 9.11437670e-01], [ 5.52846755e-01, 8.96956307e-01], [ 5.66886964e-01, 8.92197888e-01], [ 5.31786730e-01, 9.11854408e-01], [ 5.53101684e-01, 8.87659912e-01], [ 5.56256184e-01, 9.05586985e-01], [ 4.91736278e-01, 8.98619549e-01], [ 5.25995483e-01, 9.04933542e-01]])
- q_subgrid_forcing_correlation(lev)float640.5452 0.8939
array([0.5452167 , 0.89389484])
- correlation(lev)float640.5452 0.8939
array([0.5452167 , 0.89389484])
- mse(lev)float649.821e-24 1.093e-27
array([9.82143520e-24, 1.09267217e-27])
- skill(lev)float640.2967 0.7989
array([0.29672359, 0.79886685])
- levPandasIndex
PandasIndex(Int64Index([1, 2], dtype='int64', name='lev'))
- timePandasIndex
PandasIndex(Float64Index([0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0], dtype='float64', name='time'))
- xPandasIndex
PandasIndex(Float64Index([ 7812.5, 23437.5, 39062.5, 54687.5, 70312.5, 85937.5, 101562.5, 117187.5, 132812.5, 148437.5, 164062.5, 179687.5, 195312.5, 210937.5, 226562.5, 242187.5, 257812.5, 273437.5, 289062.5, 304687.5, 320312.5, 335937.5, 351562.5, 367187.5, 382812.5, 398437.5, 414062.5, 429687.5, 445312.5, 460937.5, 476562.5, 492187.5, 507812.5, 523437.5, 539062.5, 554687.5, 570312.5, 585937.5, 601562.5, 617187.5, 632812.5, 648437.5, 664062.5, 679687.5, 695312.5, 710937.5, 726562.5, 742187.5, 757812.5, 773437.5, 789062.5, 804687.5, 820312.5, 835937.5, 851562.5, 867187.5, 882812.5, 898437.5, 914062.5, 929687.5, 945312.5, 960937.5, 976562.5, 992187.5], dtype='float64', name='x'))
- yPandasIndex
PandasIndex(Float64Index([ 7812.5, 23437.5, 39062.5, 54687.5, 70312.5, 85937.5, 101562.5, 117187.5, 132812.5, 148437.5, 164062.5, 179687.5, 195312.5, 210937.5, 226562.5, 242187.5, 257812.5, 273437.5, 289062.5, 304687.5, 320312.5, 335937.5, 351562.5, 367187.5, 382812.5, 398437.5, 414062.5, 429687.5, 445312.5, 460937.5, 476562.5, 492187.5, 507812.5, 523437.5, 539062.5, 554687.5, 570312.5, 585937.5, 601562.5, 617187.5, 632812.5, 648437.5, 664062.5, 679687.5, 695312.5, 710937.5, 726562.5, 742187.5, 757812.5, 773437.5, 789062.5, 804687.5, 820312.5, 835937.5, 851562.5, 867187.5, 882812.5, 898437.5, 914062.5, 929687.5, 945312.5, 960937.5, 976562.5, 992187.5], dtype='float64', name='y'))
- pyqg:L :
- 1000000.0
- pyqg:M :
- 4096
- pyqg:W :
- 1000000.0
- pyqg:beta :
- 1.5e-11
- pyqg:del2 :
- 0.8
- pyqg:delta :
- 0.25
- pyqg:dt :
- 3600.0
- pyqg:filterfac :
- 23.6
- pyqg:nk :
- 33
- pyqg:nl :
- 64
- pyqg:ntd :
- 1
- pyqg:nx :
- 64
- pyqg:ny :
- 64
- pyqg:nz :
- 2
- pyqg:rd :
- 15000.0
- pyqg:rek :
- 5.787e-07
- pyqg:taveint :
- 86400.0
- pyqg:tavestart :
- 315360000.0
- pyqg:tc :
- 0
- pyqg:tmax :
- 1576800000.0
- pyqg:twrite :
- 1000.0
- reference :
- https://pyqg.readthedocs.io/en/latest/index.html
- title :
- pyqg: Python Quasigeostrophic Model
plt.figure(figsize=(7.6,6)).suptitle("Offline performance")
for z in [0,1]:
plt.subplot(2,2,z+1,title=f"{['Upper','Lower'][z]} layer")
imshow(preds1.q_subgrid_forcing_spatial_correlation.isel(lev=z))
if z: colorbar("Correlation")
for z in [0,1]:
plt.subplot(2,2,z+3)
imshow(preds1.q_subgrid_forcing_spatial_skill.isel(lev=z))
if z: colorbar("$R^2$")
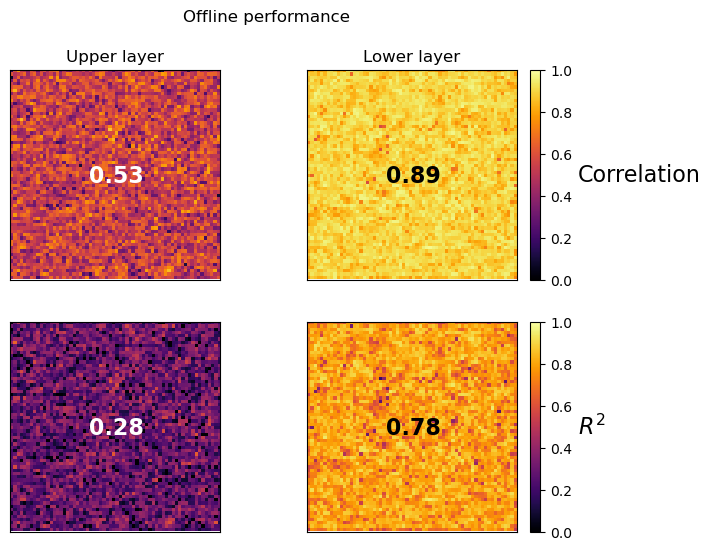
We can improve these offline metrics by training our FCNN on more datasets. Below, we feed another dataset into each model, extract the input and target features from the dataset, and standardize by calling fit()
which also then initiates a training session on the inputs and targets.
training_ds2 = xr.open_zarr('/home/jovyan/datasets/eddy/op2/ds3').load()
for m in param.models:
new_inputs = m.extract_inputs(training_ds2)
new_targets = m.extract_targets(training_ds2)
m.fit(new_inputs, new_targets, rescale=True)
We then run predictions again with the same, initial test dataset on the parameterization after its been trained on another dataset.
preds2 = param.test_offline(test_ds)
preds2
<pyqg_parameterization_benchmarks.neural_networks.FCNNParameterization object at 0x7fbff29e1d50>
<xarray.Dataset> Dimensions: (time: 87, lev: 2, y: 64, x: 64) Coordinates: * lev (lev) int64 1 2 * time (time) float64 0.0 0.0 ... 0.0 0.0 * x (x) float64 7.812e+03 ... 9.922e+05 * y (y) float64 7.812e+03 ... 9.922e+05 Data variables: (12/14) q_subgrid_forcing (time, lev, y, x) float64 5.552e-... q_subgrid_forcing_predictions (time, lev, y, x) float64 -6.373e... q_subgrid_forcing_spatial_mse (lev, y, x) float64 7.614e-24 ...... q_subgrid_forcing_temporal_mse (time, lev) float64 3.353e-27 ...... q_subgrid_forcing_mse (lev) float64 4.449e-24 8.302e-28 q_subgrid_forcing_spatial_skill (lev, y, x) float64 0.3996 ... 0.536 ... ... q_subgrid_forcing_spatial_correlation (lev, y, x) float64 0.7024 ... 0.... q_subgrid_forcing_temporal_correlation (time, lev) float64 -0.006284 ...... q_subgrid_forcing_correlation (lev) float64 0.8257 0.9204 correlation (lev) float64 0.8257 0.9204 mse (lev) float64 4.449e-24 8.302e-28 skill (lev) float64 0.6814 0.8472 Attributes: (12/23) pyqg:L: 1000000.0 pyqg:M: 4096 pyqg:W: 1000000.0 pyqg:beta: 1.5e-11 pyqg:del2: 0.8 pyqg:delta: 0.25 ... ... pyqg:tavestart: 315360000.0 pyqg:tc: 0 pyqg:tmax: 1576800000.0 pyqg:twrite: 1000.0 reference: https://pyqg.readthedocs.io/en/latest/index.html title: pyqg: Python Quasigeostrophic Model
- time: 87
- lev: 2
- y: 64
- x: 64
- lev(lev)int641 2
- long_name :
- vertical levels
array([1, 2])
- time(time)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- long_name :
- model time
- units :
- s
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
- x(x)float647.812e+03 2.344e+04 ... 9.922e+05
- long_name :
- real space grid points in the x direction
- units :
- grid point
array([ 7812.5, 23437.5, 39062.5, 54687.5, 70312.5, 85937.5, 101562.5, 117187.5, 132812.5, 148437.5, 164062.5, 179687.5, 195312.5, 210937.5, 226562.5, 242187.5, 257812.5, 273437.5, 289062.5, 304687.5, 320312.5, 335937.5, 351562.5, 367187.5, 382812.5, 398437.5, 414062.5, 429687.5, 445312.5, 460937.5, 476562.5, 492187.5, 507812.5, 523437.5, 539062.5, 554687.5, 570312.5, 585937.5, 601562.5, 617187.5, 632812.5, 648437.5, 664062.5, 679687.5, 695312.5, 710937.5, 726562.5, 742187.5, 757812.5, 773437.5, 789062.5, 804687.5, 820312.5, 835937.5, 851562.5, 867187.5, 882812.5, 898437.5, 914062.5, 929687.5, 945312.5, 960937.5, 976562.5, 992187.5])
- y(y)float647.812e+03 2.344e+04 ... 9.922e+05
- long_name :
- real space grid points in the y direction
- units :
- grid point
array([ 7812.5, 23437.5, 39062.5, 54687.5, 70312.5, 85937.5, 101562.5, 117187.5, 132812.5, 148437.5, 164062.5, 179687.5, 195312.5, 210937.5, 226562.5, 242187.5, 257812.5, 273437.5, 289062.5, 304687.5, 320312.5, 335937.5, 351562.5, 367187.5, 382812.5, 398437.5, 414062.5, 429687.5, 445312.5, 460937.5, 476562.5, 492187.5, 507812.5, 523437.5, 539062.5, 554687.5, 570312.5, 585937.5, 601562.5, 617187.5, 632812.5, 648437.5, 664062.5, 679687.5, 695312.5, 710937.5, 726562.5, 742187.5, 757812.5, 773437.5, 789062.5, 804687.5, 820312.5, 835937.5, 851562.5, 867187.5, 882812.5, 898437.5, 914062.5, 929687.5, 945312.5, 960937.5, 976562.5, 992187.5])
- q_subgrid_forcing(time, lev, y, x)float645.552e-17 3.096e-16 ... -2.656e-14
array([[[[ 5.55179776e-17, 3.09560834e-16, -1.66063278e-16, ..., 5.13362079e-17, -1.20823996e-17, 4.36760085e-17], [ 6.95917707e-17, -2.92396179e-16, 2.26111701e-16, ..., 9.56804274e-17, 9.32421941e-17, -2.13893152e-16], [-5.62658759e-17, -2.31359958e-16, 1.52806560e-16, ..., -1.66016465e-16, 1.91860586e-16, -1.41295930e-16], ..., [ 1.09645570e-16, 1.06962694e-16, -4.35254919e-17, ..., -2.47803644e-16, 1.25878311e-16, -1.33889214e-16], [ 3.40285926e-17, 9.33771833e-17, -6.26269462e-17, ..., 7.91205656e-17, 1.39150640e-16, -2.52517433e-16], [-6.93781564e-17, 5.06652452e-17, 2.45310189e-17, ..., -1.31091093e-17, -1.69117439e-16, 2.75261631e-16]], [[ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00, ..., 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], ... [-2.30867925e-11, -1.00058652e-11, 1.60266166e-12, ..., 1.06329297e-11, -1.67707475e-11, 5.95654608e-12], [ 2.53160477e-11, -2.71636402e-12, -2.98504797e-12, ..., 7.72276122e-14, 3.36663902e-12, -2.39130571e-11], [-2.41466025e-11, 1.51891312e-11, -1.63883657e-12, ..., -8.46028372e-12, 4.47933826e-13, 1.53905702e-11]], [[-1.45171667e-14, 5.74727108e-15, 8.18115069e-15, ..., 6.96897006e-15, 2.09970188e-14, 2.00784809e-14], [ 2.21630557e-14, 1.48563503e-14, 1.05098070e-15, ..., -2.64058250e-14, -4.40439393e-14, 9.04110287e-15], [ 6.62723099e-15, 1.59673282e-14, -1.70590056e-14, ..., -7.70482601e-14, -5.32238995e-14, 5.43393561e-14], ..., [-4.39536859e-14, -2.78042104e-14, -1.12086452e-13, ..., 1.88676590e-13, 5.59096073e-15, 3.58463440e-14], [-3.59522708e-14, -2.33749362e-14, 5.56709525e-15, ..., 9.79367839e-14, 1.72077204e-14, -5.29816496e-14], [ 1.10142620e-14, -3.08420529e-15, -1.41550306e-14, ..., -5.21726218e-14, -3.64078037e-14, -2.65578732e-14]]]])
- q_subgrid_forcing_predictions(time, lev, y, x)float64-6.373e-14 3.503e-13 ... -2.502e-14
array([[[[-6.37312943e-14, 3.50281002e-13, 3.50865712e-13, ..., 3.49989298e-13, 3.49838675e-13, 2.22366491e-13], [-1.47217674e-13, -5.96449169e-17, 5.72207263e-15, ..., 7.06147122e-16, -1.54410570e-15, -2.15270537e-13], [-1.47465780e-13, -4.88798044e-16, 5.39005562e-15, ..., 9.05262227e-16, -7.52782109e-16, -2.14890714e-13], ..., [-1.47205016e-13, -8.60647308e-16, 4.80042921e-15, ..., 2.07315761e-15, 7.99691376e-16, -2.14257147e-13], [-1.47415798e-13, 2.17222010e-16, 5.71579823e-15, ..., -1.11046432e-15, -2.32519523e-15, -2.15120632e-13], [ 9.97822701e-14, 1.77073552e-13, 1.77793354e-13, ..., 1.74267610e-13, 1.73478717e-13, -2.09726899e-14]], [[-8.03698742e-15, -8.35050554e-16, -8.11785577e-16, ..., -7.15632726e-16, -7.19563435e-16, -3.55856879e-15], [-1.48936344e-15, -5.43582534e-17, -6.15028969e-17, ..., 3.82863789e-17, 2.10111965e-17, -3.02127271e-15], [-1.49056909e-15, -5.65148390e-17, -6.21799078e-17, ..., 4.37975624e-17, 2.70886728e-17, -3.02015654e-15], ... [-1.74845850e-11, -4.60933852e-12, 5.33138681e-12, ..., 8.26404223e-12, -1.58647973e-11, 8.55642814e-12], [ 1.24904869e-11, -8.00758006e-13, -5.16374053e-12, ..., -3.76603393e-12, -3.64864736e-12, -1.28593195e-11], [-7.36506342e-12, 4.96642701e-12, 1.05324755e-12, ..., -5.29213132e-12, 2.75801387e-12, 7.64553091e-12]], [[-4.71885485e-15, -9.97092881e-15, -3.05749565e-15, ..., -2.99112270e-14, 3.28555610e-16, 2.52408703e-15], [ 1.14348779e-14, 1.13243587e-14, 5.27680350e-15, ..., -2.25102665e-14, -2.64358339e-14, 2.50284069e-15], [ 1.88125171e-14, 1.16914115e-15, -1.25105013e-14, ..., -7.53754088e-14, -4.71361164e-14, 5.52450177e-15], ..., [-2.51010404e-14, -2.02056864e-14, -8.37581144e-14, ..., 2.38349081e-13, 6.14782680e-14, -1.43863845e-15], [-3.19275924e-14, -1.35323424e-14, -4.01123497e-14, ..., 6.81415373e-14, -6.43595623e-14, -4.27722873e-14], [-1.06617879e-15, -4.24342966e-15, -2.75298456e-15, ..., -3.50710807e-14, -5.11121568e-14, -2.50232371e-14]]]])
- q_subgrid_forcing_spatial_mse(lev, y, x)float647.614e-24 7.309e-24 ... 1.344e-27
array([[[7.61391866e-24, 7.30901029e-24, 5.34501980e-24, ..., 8.60328099e-24, 5.06663208e-24, 9.39217882e-24], [5.19952311e-24, 4.00676386e-24, 4.42360565e-24, ..., 4.06760255e-24, 4.34494102e-24, 5.77378708e-24], [8.34339306e-24, 3.98382966e-24, 4.33452639e-24, ..., 4.24087932e-24, 4.92361206e-24, 5.98457877e-24], ..., [6.00388320e-24, 4.98484686e-24, 4.94458142e-24, ..., 5.27041960e-24, 3.10301850e-24, 9.35887701e-24], [7.34156474e-24, 4.70310396e-24, 3.39228470e-24, ..., 4.45994162e-24, 4.33162326e-24, 1.21313193e-23], [1.38586608e-23, 1.18425082e-23, 6.49575957e-24, ..., 5.16309034e-24, 4.55619074e-24, 9.35532616e-24]], [[1.15115199e-27, 1.23004070e-27, 9.56535513e-28, ..., 1.60873748e-27, 1.30943809e-27, 9.90123530e-28], [7.53211514e-28, 9.03309528e-28, 7.36072967e-28, ..., 5.89182100e-28, 7.33269097e-28, 8.14536508e-28], [1.19800666e-27, 6.59822678e-28, 9.44839439e-28, ..., 1.14296937e-27, 9.70356355e-28, 1.02228310e-27], ..., [9.49864368e-28, 7.66325490e-28, 9.77181973e-28, ..., 1.21646016e-27, 1.62390802e-27, 1.37205249e-27], [1.09382969e-27, 5.47104944e-28, 9.20062088e-28, ..., 1.16297670e-27, 9.84232674e-28, 1.37993636e-27], [1.80413545e-27, 1.38050720e-27, 1.26461595e-27, ..., 8.36535085e-28, 9.58474650e-28, 1.34440868e-27]]])
- q_subgrid_forcing_temporal_mse(time, lev)float643.353e-27 2.617e-31 ... 1.049e-27
array([[3.35280436e-27, 2.61654088e-31], [3.41090429e-27, 2.30724916e-31], [3.35447833e-27, 2.32668844e-31], [3.38882324e-27, 2.28683690e-31], [3.36900257e-27, 2.32479466e-31], [3.36402205e-27, 2.34035800e-31], [3.31230832e-27, 2.38582887e-31], [3.30179045e-27, 2.38102133e-31], [3.29884187e-27, 2.29538154e-31], [3.44871528e-27, 2.12896345e-31], [3.47439240e-27, 2.18090179e-31], [3.46765501e-27, 2.77243407e-31], [3.64878533e-27, 3.68125179e-31], [3.94093025e-27, 5.04093717e-31], [4.31297999e-27, 6.45737915e-31], [4.61189726e-27, 7.62503138e-31], [5.48228122e-27, 1.19055784e-30], [8.99562125e-27, 2.30000932e-30], [1.49465568e-26, 5.00726471e-30], [2.78485568e-26, 8.08905076e-30], ... [5.50275161e-24, 8.14372776e-28], [6.45787631e-24, 1.11110798e-27], [5.94207781e-24, 1.07637148e-27], [5.63696871e-24, 9.09156423e-28], [5.40644172e-24, 8.87445717e-28], [4.89351293e-24, 7.27525529e-28], [5.48207899e-24, 8.34859060e-28], [5.36295753e-24, 8.84959813e-28], [5.39410297e-24, 7.94352357e-28], [5.66579708e-24, 1.06280106e-27], [5.53239659e-24, 8.21243860e-28], [5.87635365e-24, 9.51701325e-28], [5.02746034e-24, 6.46549667e-28], [4.52134368e-24, 7.43758560e-28], [4.57740945e-24, 5.82092893e-28], [4.05453920e-24, 6.64761254e-28], [5.52405061e-24, 8.76783231e-28], [5.86507360e-24, 8.85188580e-28], [4.85171915e-24, 8.25486639e-28], [5.89509085e-24, 1.04909341e-27]])
- q_subgrid_forcing_mse(lev)float644.449e-24 8.302e-28
array([4.44891713e-24, 8.30246766e-28])
- q_subgrid_forcing_spatial_skill(lev, y, x)float640.3996 0.656 ... 0.8052 0.536
array([[[0.39961466, 0.65595429, 0.77478466, ..., 0.52910063, 0.5787799 , 0.34112525], [0.7073036 , 0.58905647, 0.62506986, ..., 0.70363446, 0.73312437, 0.62542269], [0.38436612, 0.71340148, 0.79706017, ..., 0.42455085, 0.52176283, 0.70343675], ..., [0.68135715, 0.78736779, 0.70079984, ..., 0.65758225, 0.82985603, 0.55038752], [0.65483155, 0.69513779, 0.6253614 , ..., 0.6311822 , 0.61261905, 0.51787762], [0.43625614, 0.59613792, 0.68587366, ..., 0.51837323, 0.68508467, 0.6166813 ]], [[0.62844415, 0.69233011, 0.80231838, ..., 0.76471653, 0.77721143, 0.70965998], [0.83815417, 0.80388074, 0.84913971, ..., 0.85815479, 0.82796171, 0.71401383], [0.71285174, 0.86035301, 0.85051771, ..., 0.91704491, 0.85023563, 0.78634852], ..., [0.82686333, 0.86109184, 0.85464733, ..., 0.87815112, 0.87053976, 0.7801975 ], [0.73849543, 0.89814731, 0.82926625, ..., 0.89966103, 0.73161155, 0.66803501], [0.60366453, 0.78197107, 0.77015729, ..., 0.82328589, 0.80524993, 0.53597259]]])
- q_subgrid_forcing_temporal_skill(time, lev)float64-1.361e+05 -2.572e+29 ... 0.8669
array([[-1.36078162e+05, -2.57150735e+29], [-1.57164438e+05, -5.74037443e+06], [-1.34626105e+05, -4.02753090e+06], [-1.25661984e+05, -2.68437862e+06], [-1.07836744e+05, -1.57197686e+06], [-8.11600112e+04, -7.82608461e+05], [-5.15133459e+04, -3.92043489e+05], [-3.88655014e+04, -2.08050364e+05], [-2.40523015e+04, -9.73989846e+04], [-1.55512182e+04, -4.77055333e+04], [-8.08445544e+03, -2.04161073e+04], [-4.17037849e+03, -1.01762218e+04], [-2.70510184e+03, -5.32826493e+03], [-1.49930337e+03, -3.08852492e+03], [-8.36304095e+02, -1.45940514e+03], [-4.56257927e+02, -6.39398260e+02], [-2.68128370e+02, -3.44789664e+02], [-1.64777088e+02, -2.06792918e+02], [-5.32448269e+01, -4.96755426e+01], [-8.04802749e-01, -2.14609926e-01], ... [ 6.83231413e-01, 8.57978596e-01], [ 6.99631370e-01, 8.55211070e-01], [ 6.95870273e-01, 8.30221471e-01], [ 6.57848153e-01, 8.54689134e-01], [ 6.65608261e-01, 8.58582209e-01], [ 6.74313227e-01, 8.64290489e-01], [ 6.69266789e-01, 8.62575479e-01], [ 6.84962075e-01, 8.43564799e-01], [ 6.72924059e-01, 8.63025773e-01], [ 6.88386354e-01, 8.34681698e-01], [ 6.85870706e-01, 8.60149933e-01], [ 6.67325611e-01, 8.33783340e-01], [ 6.72680148e-01, 8.77164638e-01], [ 6.69491201e-01, 8.49025580e-01], [ 6.99363078e-01, 8.37545764e-01], [ 6.82778347e-01, 8.74071946e-01], [ 6.71138998e-01, 8.41184202e-01], [ 7.09297877e-01, 8.60199790e-01], [ 6.79327258e-01, 8.54994153e-01], [ 7.01079014e-01, 8.66921196e-01]])
- q_subgrid_forcing_skill(lev)float640.6814 0.8472
array([0.68142961, 0.84717269])
- q_subgrid_forcing_spatial_correlation(lev, y, x)float640.7024 0.8563 ... 0.8988 0.7366
array([[[0.70235274, 0.85629677, 0.90244659, ..., 0.77398246, 0.76776424, 0.59643217], [0.86099644, 0.79527845, 0.81307245, ..., 0.85541466, 0.86101975, 0.79603831], [0.62128643, 0.84576295, 0.89196144, ..., 0.70401109, 0.73124523, 0.86063855], ..., [0.84716681, 0.8873431 , 0.83738297, ..., 0.81916713, 0.91201731, 0.76684458], [0.85636391, 0.83915449, 0.79184124, ..., 0.79998649, 0.79280786, 0.7645673 ], [0.753792 , 0.81737101, 0.83519929, ..., 0.7157449 , 0.85835347, 0.85714196]], [[0.81207564, 0.83156262, 0.90642131, ..., 0.91859461, 0.90007401, 0.88763576], [0.92451885, 0.90060115, 0.92200646, ..., 0.92928599, 0.90979325, 0.87698267], [0.84910883, 0.92878628, 0.92290734, ..., 0.96361811, 0.92227743, 0.90841662], ..., [0.92243657, 0.92902455, 0.9243516 , ..., 0.93732393, 0.94776692, 0.90444873], [0.87296159, 0.95011004, 0.91280135, ..., 0.95439666, 0.86741415, 0.83992045], [0.8773976 , 0.9110585 , 0.89170093, ..., 0.91324619, 0.89878153, 0.7365597 ]]])
- q_subgrid_forcing_temporal_correlation(time, lev)float64-0.006284 nan ... 0.8373 0.9311
array([[-6.28374542e-03, nan], [ 1.92495150e-03, -1.72375436e-02], [ 1.13176701e-02, -9.77320887e-03], [ 7.80395516e-03, -7.43350637e-03], [ 3.20768120e-03, -8.19112537e-03], [ 1.47451721e-03, 1.79418913e-03], [ 5.99960411e-03, 6.83054643e-03], [ 1.12085088e-03, -1.18528605e-02], [ 4.24546537e-03, -3.80501938e-02], [ 6.94131836e-03, -3.76010457e-02], [ 1.34454721e-03, -2.60765247e-02], [-5.07212767e-03, -2.96678457e-02], [-1.89647742e-04, -3.95527001e-02], [ 8.41438849e-03, -3.00149705e-02], [-4.00992866e-04, -1.89445186e-02], [ 2.64568284e-03, -1.73248200e-02], [ 1.31344692e-02, -3.70348544e-03], [ 1.78875151e-02, 9.79219684e-03], [ 4.90860971e-02, 8.04511761e-02], [ 5.58437252e-01, 5.66857055e-01], ... [ 8.26935424e-01, 9.26330398e-01], [ 8.36799730e-01, 9.24819191e-01], [ 8.34338789e-01, 9.11290387e-01], [ 8.11142180e-01, 9.24589557e-01], [ 8.15908966e-01, 9.26659057e-01], [ 8.22049824e-01, 9.30009197e-01], [ 8.18838831e-01, 9.28756219e-01], [ 8.28619597e-01, 9.18580422e-01], [ 8.20621396e-01, 9.28992217e-01], [ 8.30487516e-01, 9.13650300e-01], [ 8.28846604e-01, 9.27443034e-01], [ 8.17113607e-01, 9.13427219e-01], [ 8.20283445e-01, 9.36570845e-01], [ 8.18583915e-01, 9.21449098e-01], [ 8.36302485e-01, 9.15227737e-01], [ 8.27338871e-01, 9.35508753e-01], [ 8.19626334e-01, 9.17329823e-01], [ 8.42214526e-01, 9.27933607e-01], [ 8.24422994e-01, 9.25327176e-01], [ 8.37319523e-01, 9.31086031e-01]])
- q_subgrid_forcing_correlation(lev)float640.8257 0.9204
array([0.82571245, 0.92042428])
- correlation(lev)float640.8257 0.9204
array([0.82571245, 0.92042428])
- mse(lev)float644.449e-24 8.302e-28
array([4.44891713e-24, 8.30246766e-28])
- skill(lev)float640.6814 0.8472
array([0.68142961, 0.84717269])
- levPandasIndex
PandasIndex(Int64Index([1, 2], dtype='int64', name='lev'))
- timePandasIndex
PandasIndex(Float64Index([0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0], dtype='float64', name='time'))
- xPandasIndex
PandasIndex(Float64Index([ 7812.5, 23437.5, 39062.5, 54687.5, 70312.5, 85937.5, 101562.5, 117187.5, 132812.5, 148437.5, 164062.5, 179687.5, 195312.5, 210937.5, 226562.5, 242187.5, 257812.5, 273437.5, 289062.5, 304687.5, 320312.5, 335937.5, 351562.5, 367187.5, 382812.5, 398437.5, 414062.5, 429687.5, 445312.5, 460937.5, 476562.5, 492187.5, 507812.5, 523437.5, 539062.5, 554687.5, 570312.5, 585937.5, 601562.5, 617187.5, 632812.5, 648437.5, 664062.5, 679687.5, 695312.5, 710937.5, 726562.5, 742187.5, 757812.5, 773437.5, 789062.5, 804687.5, 820312.5, 835937.5, 851562.5, 867187.5, 882812.5, 898437.5, 914062.5, 929687.5, 945312.5, 960937.5, 976562.5, 992187.5], dtype='float64', name='x'))
- yPandasIndex
PandasIndex(Float64Index([ 7812.5, 23437.5, 39062.5, 54687.5, 70312.5, 85937.5, 101562.5, 117187.5, 132812.5, 148437.5, 164062.5, 179687.5, 195312.5, 210937.5, 226562.5, 242187.5, 257812.5, 273437.5, 289062.5, 304687.5, 320312.5, 335937.5, 351562.5, 367187.5, 382812.5, 398437.5, 414062.5, 429687.5, 445312.5, 460937.5, 476562.5, 492187.5, 507812.5, 523437.5, 539062.5, 554687.5, 570312.5, 585937.5, 601562.5, 617187.5, 632812.5, 648437.5, 664062.5, 679687.5, 695312.5, 710937.5, 726562.5, 742187.5, 757812.5, 773437.5, 789062.5, 804687.5, 820312.5, 835937.5, 851562.5, 867187.5, 882812.5, 898437.5, 914062.5, 929687.5, 945312.5, 960937.5, 976562.5, 992187.5], dtype='float64', name='y'))
- pyqg:L :
- 1000000.0
- pyqg:M :
- 4096
- pyqg:W :
- 1000000.0
- pyqg:beta :
- 1.5e-11
- pyqg:del2 :
- 0.8
- pyqg:delta :
- 0.25
- pyqg:dt :
- 3600.0
- pyqg:filterfac :
- 23.6
- pyqg:nk :
- 33
- pyqg:nl :
- 64
- pyqg:ntd :
- 1
- pyqg:nx :
- 64
- pyqg:ny :
- 64
- pyqg:nz :
- 2
- pyqg:rd :
- 15000.0
- pyqg:rek :
- 5.787e-07
- pyqg:taveint :
- 86400.0
- pyqg:tavestart :
- 315360000.0
- pyqg:tc :
- 0
- pyqg:tmax :
- 1576800000.0
- pyqg:twrite :
- 1000.0
- reference :
- https://pyqg.readthedocs.io/en/latest/index.html
- title :
- pyqg: Python Quasigeostrophic Model
plt.figure(figsize=(7.6,6)).suptitle("Offline performance")
for z in [0,1]:
plt.subplot(2,2,z+1,title=f"{['Upper','Lower'][z]} layer")
imshow(preds2.q_subgrid_forcing_spatial_correlation.isel(lev=z))
if z: colorbar("Correlation")
for z in [0,1]:
plt.subplot(2,2,z+3)
imshow(preds2.q_subgrid_forcing_spatial_skill.isel(lev=z))
if z: colorbar("$R^2$")
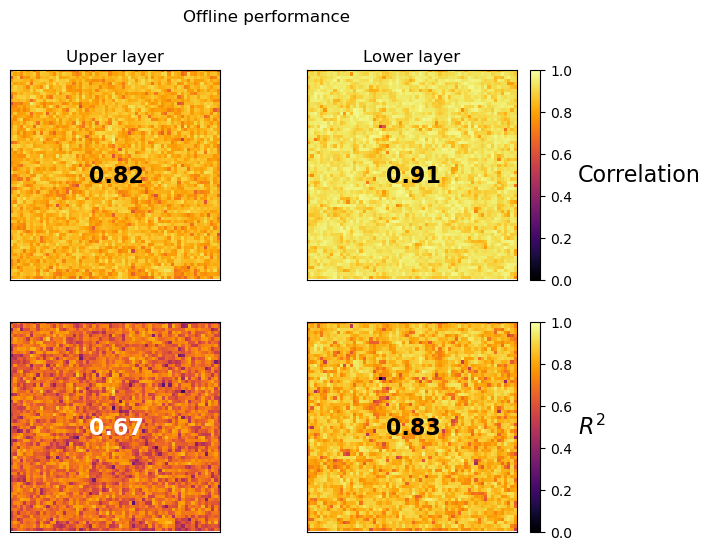
As shown, our computed metrics improved with additional training. We then save our newly trained models to the model directory path for our parameterization in order to keep the updated trained state of the models.
param.models[0].save('/home/jovyan/models/fcnn_qu_to_Sq2/models/0')
param.models[1].save('/home/jovyan/models/fcnn_qu_to_Sq2/models/1')
We can then reload the FCNN parameterization in future use cases by instantiating a new FCNNParameterization
instance and passing in the directory path at which the parameterization is saved, as follows:
param = FCNNParameterization('/home/jovyan/models/fcnn_qu_to_Sq2')